Building a React App with Firebase Authentication using AuthContext
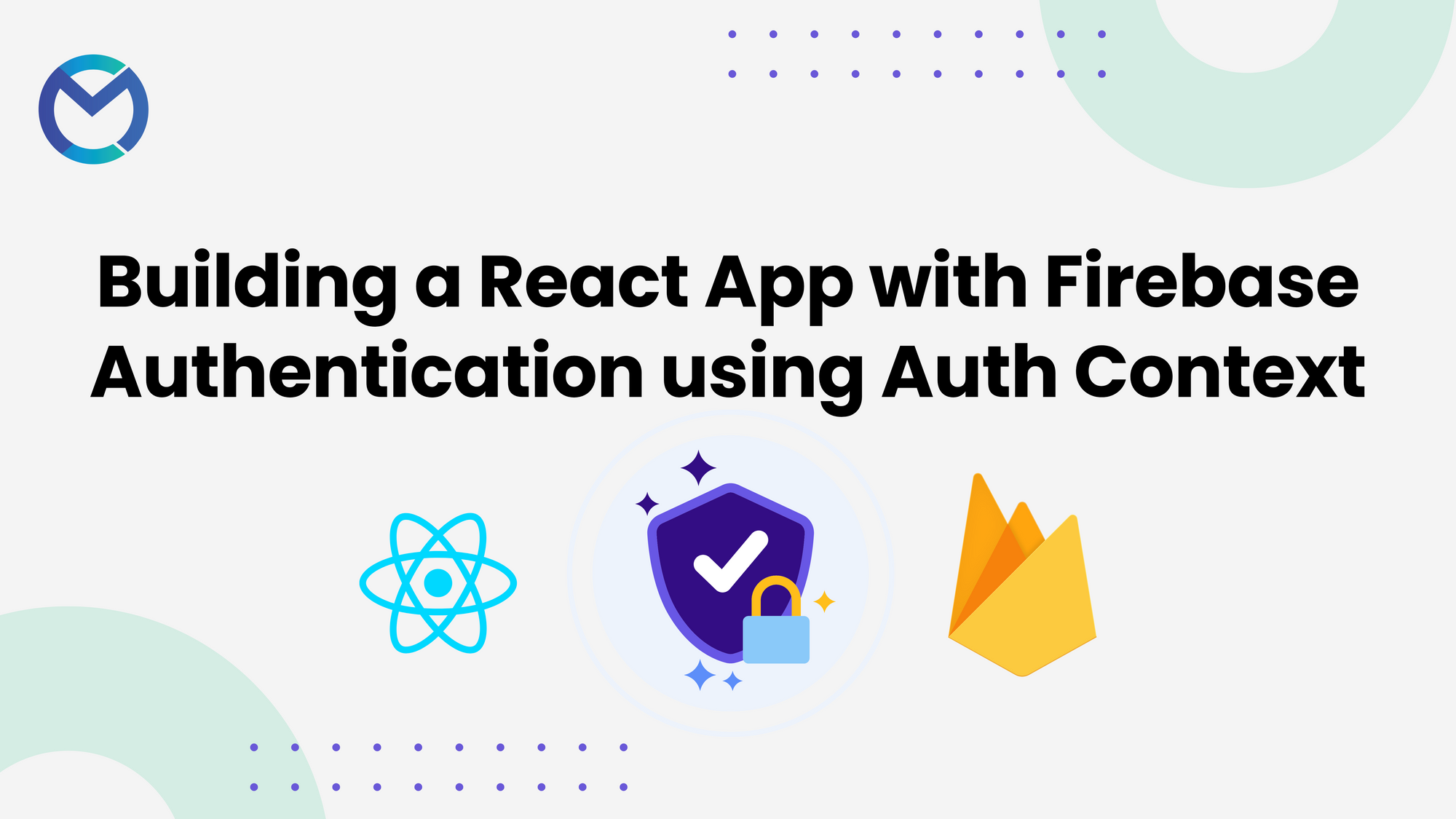
Introduction
In the rapidly evolving landscape of web applications, ensuring secure user authentication is paramount. It forms the foundation of user trust, safeguarding sensitive information and protecting against unauthorized access. Robust authentication mechanisms are crucial for preventing data breaches and maintaining the integrity of user accounts.
Simplifying the authentication process
Firebase, a comprehensive mobile and web application development platform, offers a seamless solution for implementing secure authentication in React applications. Its integration with React simplifies the implementation process, providing developers with a reliable and scalable authentication infrastructure. Firebase also brings the advantage of real-time data synchronization, making it an ideal choice for building dynamic and responsive web applications.
Technologies Used
The chosen technology stack, comprising React, Firebase, and Material-UI, synergizes to create a powerful and efficient development environment.
- React: Known for its declarative and component-based structure, React enables the creation of interactive and reusable user interfaces. Its virtual DOM ensures optimal performance by minimizing unnecessary updates.
- Firebase: A cloud-based platform, Firebase, provides a comprehensive set of tools for backend services, including real-time databases, cloud functions, and, crucially, authentication services.
- Material-UI: A React UI framework, Material-UI offers a collection of pre-designed React components following the Material Design principles. It facilitates the creation of aesthetically pleasing and responsive user interfaces.
Advantages
- Efficiency and Productivity: React's component-based architecture streamlines development and promotes code reusability. This, combined with Firebase's backend services, accelerates the development lifecycle.
- Real-time Updates: Firebase's real-time database allows for instantaneous updates, ensuring users experience dynamic and responsive applications.
- Consistent Design: Material-UI's design system promotes a consistent and visually appealing user interface, enhancing the overall user experience.
Building the React App
Firebase Setup
- Firebase is initialized using the
firebaseConfig
provided in../config
. - Firebase Authentication and Firestore are configured.
if (!firebase.apps.length) {
firebase.initializeApp(firebaseConfig);
firebase.firestore();
}
Initial State and Reducer
- The initial state for authentication is set using
initialState
. - The
reducer
function is responsible for handling state changes.
const initialState = {
isAuthenticated: false,
isInitialized: false,
user: null
};
const reducer = (state, action) => {
if (action.type === 'INITIALISE') {
const { isAuthenticated, user } = action.payload;
return {
...state,
isAuthenticated,
isInitialized: true,
user
};
}
return state;
};
AuthContext and AuthProvider
AuthContext
is created usingcreateContext()
with default values and methods.- The
AuthProvider
component manages authentication state usinguseReducer
anduseEffect
.
const AuthContext = createContext({
...initialState,
method: 'firebase',
login: () => Promise.resolve(),
register: () => Promise.resolve(),
loginWithGoogle: () => Promise.resolve(),
loginWithFaceBook: () => Promise.resolve(),
loginWithTwitter: () => Promise.resolve(),
logout: () => Promise.resolve()
});
function AuthProvider({ children }) {
// ... (explained below)
}
useEffect
listens for changes in the user's authentication state usingonAuthStateChanged
.- When a user is authenticated, their data is fetched from Firestore.
useEffect(() =>
firebase.auth().onAuthStateChanged((user) => {
// ... (explained below)
}), [dispatch]);
Authentication Functions
- Functions for login, registration, and social media logins using Firebase authentication methods.
const login = (email, password) => firebase.auth().signInWithEmailAndPassword(email, password);
const loginWithGoogle = () => {
const provider = new firebase.auth.GoogleAuthProvider();
return firebase.auth().signInWithPopup(provider);
};
// Similar functions for Facebook and Twitter logins
const register = (email, password, firstName, lastName) =>
firebase
.auth()
.createUserWithEmailAndPassword(email, password)
.then((res) => {
firebase
.firestore()
.collection('users')
.doc(res.user.uid)
.set({
uid: res.user.uid,
email,
displayName: `${firstName} ${lastName}`
});
});
User Profile Handling
- User profile data is fetched from Firestore and stored in the
profile
state.
const [profile, setProfile] = useState(null);
useEffect(() => {
// ... (explained below)
}, [dispatch]);
Context Values
- The context provider wraps the entire application, providing authentication state, user details, and methods.
const auth = { ...state.user };
<AuthContext.Provider
value={{
...state,
method: 'firebase',
user: {
// ... (explained below)
},
login,
register,
loginWithGoogle,
loginWithFaceBook,
loginWithTwitter,
logout,
resetPassword
}}
>
{children}
</AuthContext.Provider>;
Using the Code
- Integrate into Your React App:
- Import the
AuthProvider
andAuthContext
into your app. - Wrap your app with
AuthProvider
to provide authentication context globally.
- Import the
import { AuthProvider, AuthContext } from './path-to-auth-provider';
function App() {
return (
<AuthProvider>
{/* Your app components */}
</AuthProvider>
);
}
- Access AuthContext Values and Methods:
- Use
useContext(AuthContext)
in any component to access the authentication context. - You can now access the authentication state, user details, and authentication methods.
- Use
import { useContext } from 'react';
import { AuthContext } from './path-to-auth-provider';
function MyComponent() {
const authContext = useContext(AuthContext);
// Access values and methods
const { isAuthenticated, user, login, register, logout, resetPassword } = authContext;
// Your component logic
}
Build Login and Register Pages
- Create components for login and register pages.
- Use the
login
,register
, and other authentication methods provided by the context.
// Example of using login function in a Login component
const handleLogin = async () => {
try {
await login(email, password);
// Handle successful login
} catch (error) {
// Handle login error
}
};
The login functionality involves using Firebase authentication methods to validate user credentials. Upon successful authentication, user data is retrieved from Firestore, populating the user's profile.
Registration Functionality
For user registration, the code utilizes Firebase's authentication service to create a new user account. Additional user data, such as the user's full name, is stored in Firestore for a more comprehensive user profile.
Social Media Logins
The integration of social media logins, including Google, Facebook, and Twitter, enhances user convenience and expands the accessibility of the authentication system.
Security Considerations
Highlighting the importance of Firebase security rules, this section emphasizes configuring rules that ensure secure user authentication. Properly configured security rules prevent unauthorized access and protect sensitive user data.
Testing the App
Suggesting tips for testing and debugging the authentication system ensures the robustness of the application. Encouraging readers to leverage the Firebase authentication console for monitoring user data provides an efficient means of tracking authentication-related activities.
GitHub Repository: https://github.com/kaviyarasumaran/Firebase-authContext
Final Thoughts
In conclusion, building a React app with Firebase Authentication using AuthContext offers a powerful and secure solution for managing user authentication in your applications.
The AuthContext, coupled with Firebase's authentication services, provides a seamless and efficient way to handle user sign-up, login and protected routes within your React app. By leveraging the capabilities of Firebase, developers can focus more on creating engaging user experiences and less on managing authentication complexities.