Building a Redux-Powered Blog with a Mock API
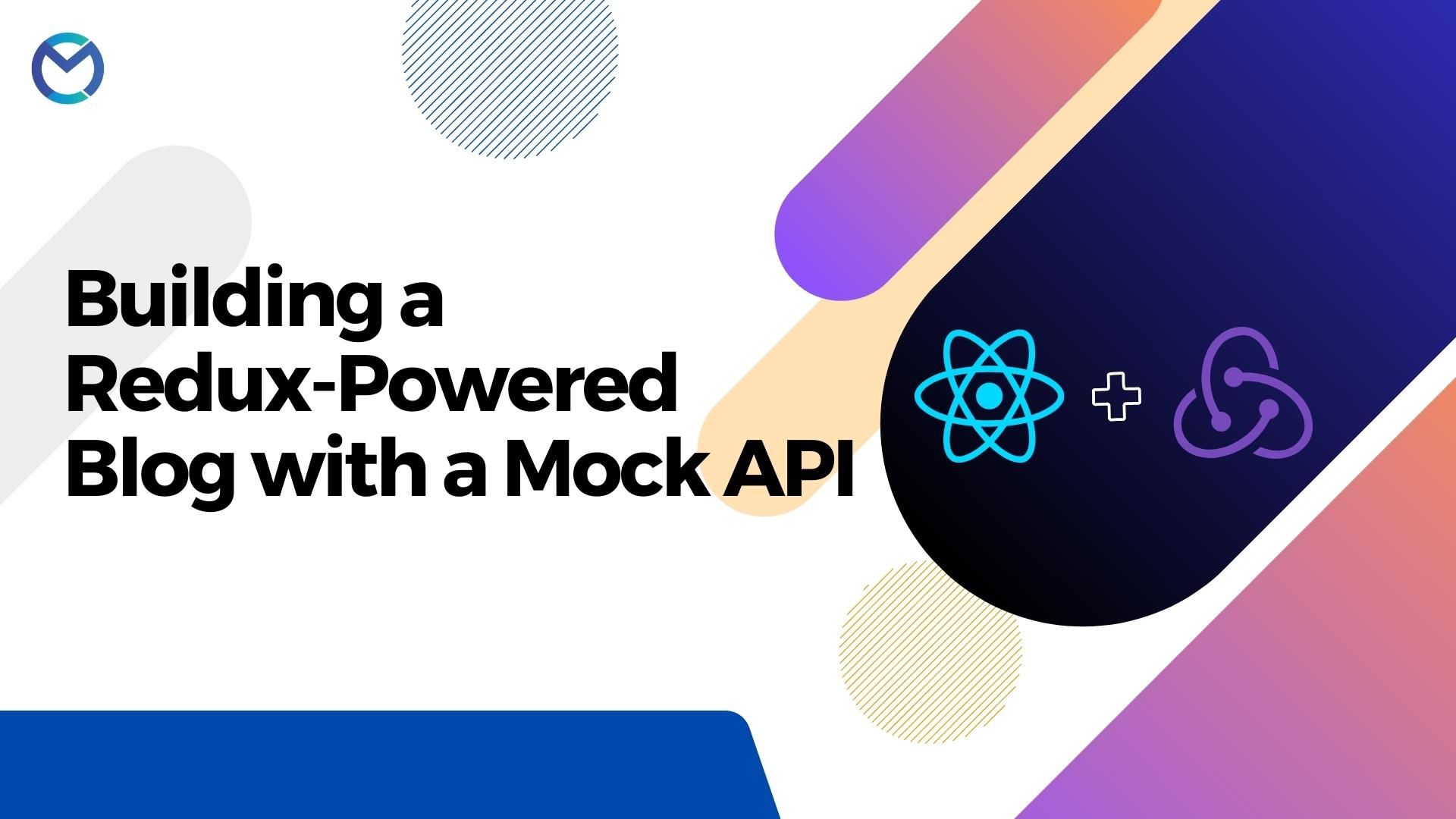
Introduction
React Redux is a powerful state management library that seamlessly integrates with React applications, offering centralized and efficient control over the application's data flow. It simplifies complex data management tasks, enhances scalability, and ensures a smooth and predictable user experience. By connecting React components to a single source of truth, React Redux empowers developers to build robust and maintainable applications with ease.
Setting Up the Redux Store
- Importing and using createSlice from Redux Toolkit This section covers the initialization of Redux using createSlice, a Redux Toolkit utility. We'll explain how it simplifies the process of creating reducers and actions.
- Initializing the Redux store with the initial state We'll discuss how the Redux store is initialized with an initial state. The initial state contains properties like isLoading, error, posts, and others that represent different aspects of the application's state.
- Defining reducers for handling different actions Here, we'll explore the concept of reducers in Redux. Reducers are functions responsible for modifying the application's state based on dispatched actions. We'll define reducers for various actions such as loading, error handling, and data retrieval.
Handling Loading States and Errors
- Implementing actions for starting and stopping loading states We'll explain how actions like startLoading and hasError are used to manage loading states. When an action is dispatched, it changes the state, indicating whether the application is in a loading state or if an error has occurred.
- Managing errors in Redux state This part will focus on how Redux handles errors. When an error occurs during an API request, it's stored in the Redux state. We'll explore how the error state is updated and displayed to the user.
Fetching Blog Posts
- Creating an action to fetch all blog posts We'll explain the action getAllPosts responsible for fetching all blog posts. It triggers an API request using Axios to retrieve the data.
- Using Axios to make API requests Here, we'll discuss the role of Axios, a popular HTTP client, in making API requests. We'll explain how it communicates with the mock API and retrieves the blog post data.
- Updating Redux state with fetched posts We'll demonstrate how the data retrieved from the API is updated in the Redux state, specifically in the posts property.
Code :
import { createSlice } from "@reduxjs/toolkit";
// utils
import axios from "../../utils/axios";
// ----------------------------------------------------------------------
const initialState = {
isLoading: false,
error: false,
posts: [],
post: null,
recentPosts: [],
hasMore: true,
index: 0,
step: 11,
};
const slice = createSlice({
name: "blog",
initialState,
reducers: {
// START LOADING
startLoading(state) {
state.isLoading = true;
},
// HAS ERROR
hasError(state, action) {
state.isLoading = false;
state.error = action.payload;
},
// GET POSTS
getPostsSuccess(state, action) {
state.isLoading = false;
state.posts = action.payload;
},
// GET POST INFINITE
getPostsInitial(state, action) {
state.isLoading = false;
state.posts = action.payload;
},
getMorePosts(state) {
const setIndex = state.index + state.step;
state.index = setIndex;
},
noHasMore(state) {
state.hasMore = false;
},
// GET POST
getPostSuccess(state, action) {
state.isLoading = false;
state.post = action.payload;
},
// GET RECENT POST
getRecentPostsSuccess(state, action) {
state.isLoading = false;
state.recentPosts = action.payload;
},
},
});
// Reducer
export default slice.reducer;
// Actions
export const { getMorePosts } = slice.actions;
// ----------------------------------------------------------------------
export function getAllPosts() {
return async (dispatch) => {
dispatch(slice.actions.startLoading());
try {
const response = await axios.get("/api/blog/posts/all");
dispatch(slice.actions.getPostsSuccess(response.data.posts));
} catch (error) {
dispatch(slice.actions.hasError(error));
}
};
}
// ----------------------------------------------------------------------
export function getPostsInitial(index, step) {
return async (dispatch) => {
dispatch(slice.actions.startLoading());
try {
const response = await axios.get("/api/blog/posts", {
params: { index, step },
});
const results = response.data.results.length;
const { maxLength } = response.data;
dispatch(slice.actions.getPostsInitial(response.data.results));
if (results >= maxLength) {
dispatch(slice.actions.noHasMore());
}
} catch (error) {
dispatch(slice.actions.hasError(error));
}
};
}
// ----------------------------------------------------------------------
export function getPost(title) {
return async (dispatch) => {
dispatch(slice.actions.startLoading());
try {
const response = await axios.get("/api/blog/post", {
params: { title },
});
dispatch(slice.actions.getPostSuccess(response.data.post));
} catch (error) {
console.error(error);
dispatch(slice.actions.hasError(error));
}
};
}
// ----------------------------------------------------------------------
export function getRecentPosts(title) {
return async (dispatch) => {
dispatch(slice.actions.startLoading());
try {
const response = await axios.get("/api/blog/posts/recent", {
params: { title },
});
dispatch(slice.actions.getRecentPostsSuccess(response.data.recentPosts));
} catch (error) {
console.error(error);
dispatch(slice.actions.hasError(error));
}
};
}
Fetching Initial Posts with Pagination
- Implementing pagination for the blog posts We'll introduce the concept of pagination and why it's crucial for large datasets. Pagination allows users to view a limited number of posts at a time.
- Defining an action to fetch initial posts with index and step parameters This section covers the getPostsInitial action, which fetches the initial set of posts using index and step parameters. The index parameter determines the starting point, and the step parameter defines the number of posts to retrieve.
- Handling pagination in the Redux state We'll explain how the pagination data, including the current index and step, is managed in the Redux state.
- dispatch(slice.actions.getRecentPostsSuccess(response.data.recentPosts)); } catch (error) { console.error(error); dispatch(slice.actions.hasError(error)); } }; }
Loading More Posts
- Explaining the logic for loading more posts We'll discuss the logic behind loading more posts as users scroll through the blog. It's a critical user experience improvement for infinite scrolling.
- Creating an action to load additional posts This section covers the getMorePosts action, which loads additional posts when users reach the end of the current list.
- Updating the Redux state to add more posts We'll show how the Redux state is updated to append newly loaded posts to the existing list.
Handling the End of Pagination
- Detecting the end of available posts Here, we'll explain how the application detects when there are no more posts to load. This ensures that the "Load More" button is appropriately disabled.
- Updating the Redux state to indicate no more posts We'll discuss how the noHasMore action is used to update the Redux state when there are no more posts to load.
- This breakdown continues in the next response to cover the remaining topics and subtopics.
Fetching Individual Blog Posts
- Creating an action to fetch a specific blog post This section covers the getPost action, which is responsible for fetching an individual blog post based on its title. We'll explain how it uses Axios to make the API request.
- Using query parameters for API requests We'll delve into the concept of query parameters in API requests and how they are used to specify which blog post to retrieve based on its title.
- Updating Redux state with the fetched blog post Here, we'll discuss how the data for the fetched blog post is updated in the Redux state, specifically in the post property.
Putting It All Together - Workflow and Data Flow
- Explaining the overall workflow of the Redux-powered blog This section provides an overview of the entire workflow, from initiating actions to updating the Redux state and rendering content in the components. It ties together all the previously discussed actions and their roles in the application's flow.
- Discussing how actions trigger state updates We'll dive into the mechanism by which actions trigger updates to the Redux state. This includes the use of reducers to modify the state in a predictable manner.
- Visualizing the data flow from API to Redux store to components Here, we'll visualize how data flows through the application, starting with API requests, updating the Redux store, and ultimately rendering in React components. Understanding this data flow is crucial for maintaining a consistent and efficient application.
Conclusion
By centralizing the application's state and actions, Redux simplifies debugging, testing, and maintaining large-scale applications. Its unidirectional data flow and time-travel debugging capabilities make it an indispensable tool for developers aiming to create efficient and scalable React applications.