CI/CD for a single branch using Azure DevOps
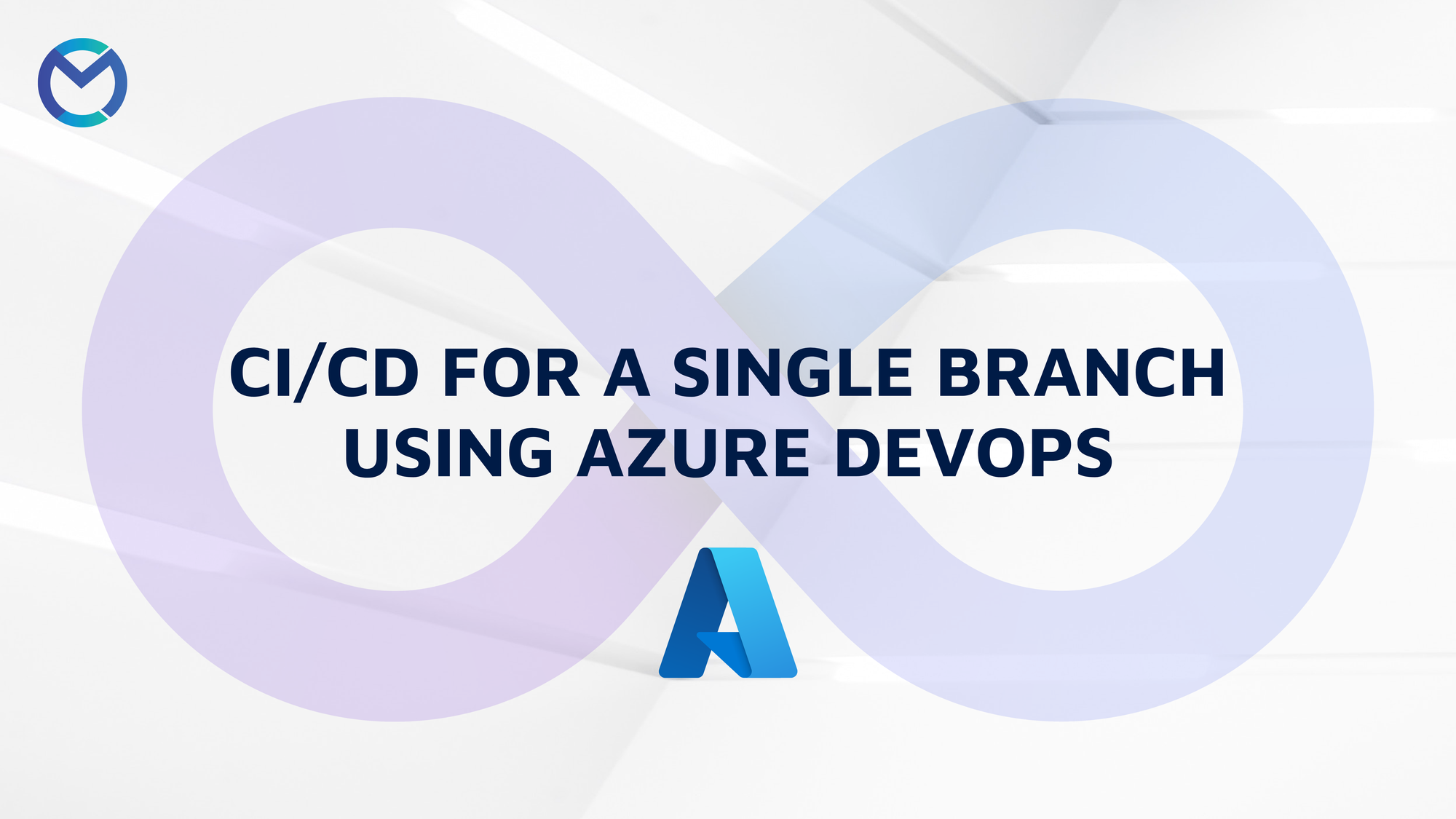
Overview
This document provides a comprehensive explanation of the Azure Pipeline script used for automating the build, test, publish, and deployment processes of a MuleSoft application. It details the stages of the pipeline, the use of environments, and the role of variable groups. Additionally, it includes the configuration of the pom.xml file used in the deployment process.
Key Concepts
What is an Azure Pipeline?
An Azure Pipeline is a set of automated steps that build, test, and deploy applications. It helps integrate and deploy code changes efficiently and reliably.
What are Environments?
Environments in Azure Pipelines represent different stages or targets where the application is deployed. They help manage deployments, track their status, and can require approvals.
What are Variable Groups?
Variable Groups are collections of variables that can be used across multiple pipelines. They provide a way to manage and share common variables (such as credentials and environment settings) in a centralized manner.
Pipeline Configuration Pool Configuration
pool :
vmlmage: ubuntu-latest
• vmlmage: Specifies the virtual machine image for the pipeline. ubuntu-latest provides the latest version of Ubuntu for the build agent.
Trigger:
Trigger
- main
- feature/ *
main: Triggers the pipeline when changes are made to the main branch.
feature/: Triggers the pipeline for any branch starting with feature/.
Variables :
variables:
name: javaVersion
value: 17
name: sandboxappName
value: {project-name}
name: ismain
value: $[eq(variables[ 'Build .SourceBranch' ] , refs/ heads/main ' ) ]
name: branchName
value: $(echo $BUILD_SOURCEBRANCH I sed 's/refs\/heads\///' )
- javaVersion: Specifies the Java version (Java 17) for the build.
- sandboxappName: The name of the MuleSoft application to be deployed.
- ismain: Boolean variable indicating if the current branch is main.
- branchName: Extracts the branch name from the source branch reference.
Variable Groups
Variable groups provide centralized management for environment-specific settings. They are used as follows:
e sandbox-variable-group: Contains variables for the Development environment.
- QA-variable-group: Contains variables for the QA environment.
- prod-variable-group: Contains variables for the Production environment.
Pipeline Stages
1. Build, Test, Publish
yaml
-stage: build_test_publish
displayName: 'Build > Tests > Publish artifact'
jobs:
- job: build_test_publish
variables:
- group: sandbox-variable-group
displayName: 'Build > Test > Publish to Exchange'
steps:
....
Purpose: Handles building the MuleSoft application, running tests, and publishing the build artifact to a Maven repository.
Key Steps:
- Checkout Code
- Set Java Version
- Configure Maven
- Increment Version
- Deploy to Exchange
Deploy to Development Environment
yaml
- stage: deploy_application_to_DEVELOPMENT
displayName: 'Deploy to DEVELOPMENT'
dependsOn: build_test_publish
jobs:
job: build_deploy_to_DEVELOPMENT
variables:
-group: sandbox-variable-group
displayName : 'Building and deploying to DEVELOPMENT Environment'
steps:
.....
Purpose: Deploys the MuleSoft application to the Development environment.
Dependencies: Runs after the successful completion of the build_test_publish stage.
Key Steps:
- Checkout Code
- Set Java Version
- Configure Maven
- Deploy to Development Environment
Deploy to QA Environment
yaml
- stage: deploy_application_to_QA
displayName : 'Deploy to QA'
jobs:
deployment : build_deploy_to_QA
displayName : 'Building and deploying to QA Environment'
environment : mulesoftqa variables:
group: QA-variable-group strategy :
runOnce:
deploy :
steps:
....
Purpose: Deploys the MuleSoft application to the QA environment.
Environment: mulesoftqa specifies that this deployment is to the QA environment.
Key Steps:
- Checkout Code
- Set Java Version
- Configure Maven
- Deploy to QA Environment
Deploy to Production Environment
yaml
-stage: deploy_application_to_PROD
displayName : 'Deploy to PROD'
condition: eq(variables[ 'Build. SourceBranch' ], ' refs/ heads/main )
-jobs:
deployment: build_deploy_to_PROD
displayName : 'Building and deploying to Production Environment'
environment: mulesoftprod variables:
group: prod-variable-group strategy :
runOnce:
deploy :
steps:
.....
Purpose: Deploys the MuleSoft application to the Production environment.
Condition: Runs only if the source branch is main.
Environment: mulesoftprod specifies that this deployment is to the Production environment.
Key Steps:
- Checkout Code
- Set Java Version
- Configure Maven
- Deploy to Production Environment
Git Tagging
yaml
- stage: git_tagging
displayName : 'Tagging main after Production deploy'
jobs:
-job: git_tagging_work
displayName : 'Git Tagging
steps:
....
Purpose: Tags the main branch in Git with the new version number after a successful Production deployment.
Condition: Runs only if the Production deployment is successful and the branch is main.
Key Steps:
- Checkout Code
- Tag Repository
- Environments and Approvals
What is an Environment?
An environment in Azure Pipelines represents a specific stage or deployment target, such as Development, QA, or Production. Environments help manage and control deployments, and they allow for setting up approvals and tracking deployment status.
Usage of Environments in the Pipeline
- Development: Used for initial deployment after building and testing. Variable group sandbox-variablegroup is used for Development-specific configurations.
- QA: Used for additional testing before Production deployment. Deployment to this environment is associated with mulesoftqa, and it may require manual approval before proceeding.
- Production: Used for final deployment. Deployment to this environment is associated with mulesoftprod, and it usually involves strict approval processes to ensure stability.
Environments help ensure that deployments are controlled and monitored, providing a structured approach to releasing updates.
pom.xml Configuration
The pom.xml file defines the project's build and deployment configurations. Below is the detailed configuration:
xml
<distributionManagement>
< repository>
<id>anypoint-exchange-v3</id>
<name>Anypoint Exchange</name>
<url>https://maven.anypoint.mu1esoft.com/api/v3/organizations/ {your organizations ID}/maven</url>
< layout>default</layout> < / repository>
</distributionManagement>
<build>
<plugins>
<plugin>
<artifactld>maven-clean-plugin</artifactld>
<version>3.2. </plugin>
<plugin>
<groupld>org.mule . tools . maven</ groupld> <artifactld>mule-maven-plugin</artifactld>
<version>{mule . maven . plugin . version}</version>
<extensions>true</extensions>
<configuration>
<cloudhub2Deployment>
<provider>{provider}</provider>
<environment>{cloudHubEnvironment}</environment>
<applicationName>{applicationName}</applicationName>
< target>{targetName}</target>
< releaseChannel>Edge</releaseChannel>
<javaVersion>17</javaVersion>
<connectedAppClientId>{connectedAppClientId}</connectedAppClientId> < connectedAppClientSecret>{connectedAppClientSecret}</connectedAppClientSecret>
<connectedAppGrantType>c1ient_credentials</connectedAppGrantType>
<replicas>1</replicas>
<vCores> .1</vCores>
< integrations>
<services>
<0bjectStoreV2>
<enabled>true</enabled>
</objectStoreV2>
< / services>
< / integrations >
<deploymentSettings>
<updateStrategy>rolling</updateStrategy>
<http>
< inbound>
<publicUrl>{publicUr1}</publicUrl>
<forwardSslSession>fa1se</forwardSslSession>
<lastMileSecuritY>fa1se</lastMileSecuritY>
</inbound>
</http>
<generateDefaultPublicUrl>true</generateDefaultPublicUrl>
< / deploymentSettings>
<properties>
<env>{env}</env>
<secure . key>{secure . key}</secure . key>
<anypoint . platform . client_id>{anypoint . platform . client_id}</anypoint . platform. client_id>
< anypoint . platform . client_secret>${anypoint . platform. client_secret</anypoint . platform. client secret >
</properties>
</cloudhub2Deployment>
<classifier>mule-application</classifier>
</configuration>
< / plugin>
</plugins>
</build>
<repositories>
< repository>
<id>anypoint-exchange-v3</id>
<name>Anypoint Exchange</name>
<url>https://maven.anypoint.mulesoft.com/api/v3/maven </url>
< layout>default</layout>
< / repository>
<repository>
<id>mulesoft-releases</id>
<name>MuleSoft Releases Repository</name>
<url>https://repository.mulesoft . org/releases/ </url>
< layout>default</layout>
< / repository>
< / repositories >
<pluginRepositories> <pluginRepository>
<id>mulesoft-releases</id>
<name>MuleSoft Releases Repository</name>
< layout>default</layout>
<url>https://repository.mulesoft . org/releases/ </url>
< snapshots>
<enabled>false</enabled>
< / snapshots>
</pluginRepository>
</pluginRepositories>
distributionManagement: Defines where to publish the build artifacts.
build: Configures the Maven plugins and deployment settings.
mule-maven-plugin: Manages deployment to CloudHub with detailed settings.
repositories: Lists the repositories used to fetch dependencies.
- anypoint-exchange-v3: Anypoint Exchange repository URL.
- mulesoft-releases: MuleSoft Releases repository URL.
pluginRepositories: Specifies where Maven plugins are fetched from.
- mulesoft-releases: MuleSoft Releases repository for plugins.
Conclusion
This Azure Pipeline script automates the process of building, testing, publishing, and deploying a MuleSoft application. By leveraging different stages and environments, the pipeline ensures that the application is thoroughly tested and deployed in a controlled manner. Variable groups are used to manage and share environment-specific settings across the pipeline, facilitating consistent and accurate deployments. The pom.xml configuration details the build and deployment specifications necessary for MuleSoft application deployments.