Creating Stunning Text Animations with Framer Motion in React MUI Part -1
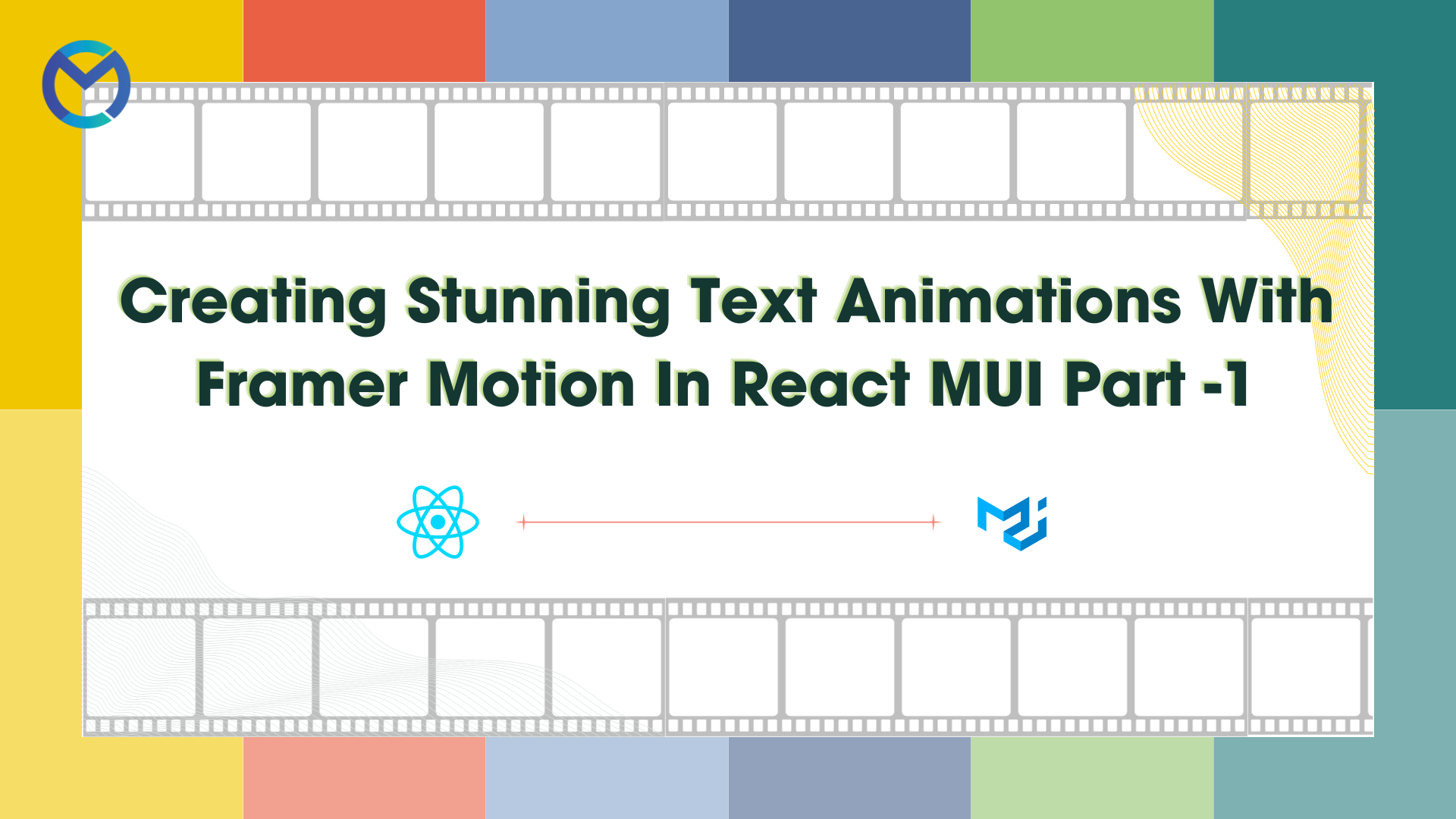
Text animations can breathe life into your React MUI (Material-UI) applications, making them more engaging and interactive. Framer Motion, a powerful animation library for React, offers a straightforward solution for adding captivating text animations to your projects. In this comprehensive guide, we'll explore how to use Framer Motion to create stunning text animations within your React MUI applications. We will cover various animation effects, such as fading and sliding, with detailed explanations and practical examples.
Getting Started with Framer Motion and React MUI
Introduction to Framer Motion
Framer Motion is a JavaScript animation library that simplifies the creation of animations in React applications. It provides a wide range of animation capabilities, including text animation, making it an excellent choice for enhancing React MUI projects.
Step 1: Setting Up a React Application
To get started, make sure you have Node.js and npm (Node Package Manager) installed on your system. If not, you can download and install them from the official website: https://nodejs.org/
Now, open your terminal and run the following command to create a new React application using Create React App:
npx create-react-app text-slide-animation-app
Step 2: Code Implementation
Inside your newly created React application directory, navigate to the src folder. In the src folder, you can create a new file named TextSlideAnimation.js to define your animation components.
Installing Framer Motion
To begin using Framer Motion, you'll need to install it in your project. We'll walk you through the installation process and ensure everything is set up correctly.
Step 2: Code Implementation
Inside your newly created React application directory, navigate to the src folder. In the src folder, you can create a new file named TextSlideAnimation.js to define your animation components.
Installing Framer Motion
To begin using Framer Motion, you'll need to install it in your project. We'll walk you through the installation process and ensure everything is set up correctly.
npm i framer-motion
npm install prop-types
Step 4: Use the Animation file
DialogAnimation.js
macOS/Linux (curl)NPMHomebrewDockerProto
import PropTypes from "prop-types";
import { motion, AnimatePresence } from "framer-motion";
import { varFadeInUp } from "./variants";
import { Dialog } from "@mui/material";
DialogAnimate.propTypes = {
open: PropTypes.bool.isRequired,
animate: PropTypes.object,
onClose: PropTypes.func,
children: PropTypes.node.isRequired,
};
export default function DialogAnimate({
open = false,
animate,
onClose,
children,
...other
}) {
return (
<AnimatePresence>
{open && (
<Dialog
fullWidth
maxWidth="xs"
open={open}
onClose={onClose}
PaperComponent={motion.div}
PaperProps={{
sx: {
borderRadius: 2,
bgcolor: "background.paper",
},
...(animate || varFadeInUp),
}}
{...other}
>
{children}
</Dialog>
)}
</AnimatePresence>
);
}
variants/fade/fadein.js
const DISTANCE = 120;
const TRANSITION_ENTER = {
duration: 0.64,
ease: [0.43, 0.13, 0.23, 0.96]
};
const TRANSITION_EXIT = {
duration: 0.48,
ease: [0.43, 0.13, 0.23, 0.96]
};
export const varFadeIn = {
initial: { opacity: 0 },
animate: { opacity: 1, transition: TRANSITION_ENTER },
exit: { opacity: 0, transition: TRANSITION_EXIT }
};
export const varFadeInUp = {
initial: { y: DISTANCE, opacity: 0 },
animate: { y: 0, opacity: 1, transition: TRANSITION_ENTER },
exit: { y: DISTANCE, opacity: 0, transition: TRANSITION_EXIT }
};
export const varFadeInLeft = {
initial: { x: -DISTANCE, opacity: 0 },
animate: { x: 0, opacity: 1, transition: TRANSITION_ENTER },
exit: { x: -DISTANCE, opacity: 0, transition: TRANSITION_EXIT }
};
export const varFadeInDown = {
initial: { y: -DISTANCE, opacity: 0 },
animate: { y: 0, opacity: 1, transition: TRANSITION_ENTER },
exit: { y: -DISTANCE, opacity: 0, transition: TRANSITION_EXIT }
};
export const varFadeInRight = {
initial: { x: DISTANCE, opacity: 0 },
animate: { x: 0, opacity: 1, transition: TRANSITION_ENTER },
exit: { x: DISTANCE, opacity: 0, transition: TRANSITION_EXIT }
};
variants/fade/fadeout.js
const DISTANCE = 120;
const TRANSITION_ENTER = {
duration: 0.64,
ease: [0.43, 0.13, 0.23, 0.96]
};
const TRANSITION_EXIT = {
duration: 0.48,
ease: [0.43, 0.13, 0.23, 0.96]
};
export const varFadeOut = {
initial: { opacity: 1 },
animate: { opacity: 0, transition: TRANSITION_ENTER },
exit: { opacity: 1, transition: TRANSITION_EXIT }
};
export const varFadeOutUp = {
initial: { y: 0, opacity: 1 },
animate: { y: -DISTANCE, opacity: 0, transition: TRANSITION_ENTER },
exit: { y: 0, opacity: 1, transition: TRANSITION_EXIT }
};
export const varFadeOutDown = {
initial: { y: 0, opacity: 1 },
animate: { y: DISTANCE, opacity: 0, transition: TRANSITION_ENTER },
exit: { y: 0, opacity: 1, transition: TRANSITION_EXIT }
};
export const varFadeOutLeft = {
initial: { x: 0, opacity: 1 },
animate: { x: -DISTANCE, opacity: 0, transition: TRANSITION_ENTER },
exit: { x: 0, opacity: 1, transition: TRANSITION_EXIT }
};
export const varFadeOutRight = {
initial: { x: 0, opacity: 1 },
animate: { x: DISTANCE, opacity: 0, transition: TRANSITION_ENTER },
exit: { x: 0, opacity: 1, transition: TRANSITION_EXIT }
};
variants/fade/index.js
export * from './FadeIn';
export * from './FadeOut';
Step 5: Use the Animation Component
import { motion } from "framer-motion";
import { Link as RouterLink } from "react-router-dom";
import { styled } from "@mui/styles";
import { Button, Box, Container, Typography, Stack } from "@mui/material";
import { PATH_DASHBOARD } from "../../../routes/paths";
import { varFadeInUp, varWrapEnter, varFadeInRight, varFadeInLeft, varFadeInDown } from "../../animate";
const RootStyle = styled(motion.div)(({ theme }) => ({
position: "relative",
backgroundColor: theme.palette.grey[200],
[theme.breakpoints.up("md")]: {
top: 0,
left: 0,
width: "100%",
height: "100vh",
display: "flex",
position: "fixed",
alignItems: "center",
},
}));
const ContentStyle = styled((props) => <Stack spacing={5} {...props} />)(
({ theme }) => ({
zIndex: 10,
maxWidth: 520,
margin: "auto",
textAlign: "center",
position: "relative",
paddingTop: theme.spacing(15),
paddingBottom: theme.spacing(15),
[theme.breakpoints.up("md")]: {
margin: "unset",
textAlign: "left",
},
})
);
const HeroImgStyle = styled(motion.img)(({ theme }) => ({
top: 0,
right: 0,
bottom: 0,
zIndex: 8,
width: "100%",
margin: "auto",
position: "absolute",
[theme.breakpoints.up("md")]: {
right: "8%",
width: "auto",
height: "70vh",
},
}));
export default function LandingHero() {
return (
<>
<RootStyle initial="initial" animate="animate" variants={varWrapEnter}>
<Box
sx={{
display: { xs: "none", sm: "none", md: "flex", lg: "flex" },
}}
>
</Box>
<Container maxWidth="lg">
<ContentStyle>
<motion.div variants={varFadeInRight}>
<Typography variant="h1" sx={{ color: "common.white" }}>
<Typography
component="span"
variant="h1"
// sx={{ color: "primary.main" }}
color={"black"}
>
"hey hi i am kavi"
</Typography>
</Typography>
</motion.div>
<motion.div variants={varFadeInLeft}>
<Typography variant="h1" sx={{ color: "common.white" }}>
<Typography
component="span"
variant="h1"
// sx={{ color: "primary.main" }}
color={"black"}
>
"hey hi i am kavi"
</Typography>
</Typography>
</motion.div>
<motion.div variants={varFadeInUp}>
<Typography variant="h1" sx={{ color: "common.white" }}>
<Typography
component="span"
variant="h1"
// sx={{ color: "primary.main" }}
color={"black"}
>
"hey hi i am kavi"
</Typography>
</Typography>
</motion.div>
<motion.div variants={varFadeInDown}>
<Typography variant="h1" sx={{ color: "common.white" }}>
<Typography
component="span"
variant="h1"
// sx={{ color: "primary.main" }}
color={"black"}
>
"hey hi i am kavi"
</Typography>
</Typography>
</motion.div>
</ContentStyle>
</Container>
</RootStyle>
<Box sx={{ height: { md: "100vh" } }} />
</>
);
}
Exploring the TextAnimate Component
Component Structure
The core of our text animations resides in the TextAnimate component. We'll start by breaking down its structure and explaining how it functions within your React MUI application.
PropTypes and Their Significance
Understanding PropTypes is crucial for maintaining code quality and preventing errors. We will explain the PropTypes used in the TextAnimate component and discuss their importance.
Typography in React MUI
Typography components play a significant role in text presentation within Material-UI. We'll explore how Typography components work and how they can be enhanced with animations.
Splitting Text into Individual Letters
To animate individual letters of text, we need to split them into separate elements. We will demonstrate how to split text into individual letters using JavaScript and React.
Implementing Text Animation
With the foundational knowledge in place, we will show you how to create a simple text animation using Framer Motion. We'll cover fading and motion effects to bring your text to life.
Fading Text Animation
Understanding the varFadeInUp Variant
The varFadeInUp variant is a key element in the fading text animation. We'll provide a detailed explanation of its properties and how it controls the animation.
Creating a Typography Component
In this section, we will guide you through creating a custom Typography component that can be animated using Framer Motion. This component will serve as the canvas for your text animations.
Utilizing motion.h1 and motion.span
To achieve smooth and stylish fading effects, we'll explain how to apply the motion.h1 and motion. span components from Framer Motion to your Typography elements.
Controlling Animation with Variants
The key to successful text animations lies in controlling them through variants. We will show you how to apply variants to your text elements and manage their properties to achieve the desired effects.
Creating Custom Text Animations & Modifying Animation Durations
Customizing the animation duration allows you to control the speed of your text animations. We'll show you how to adjust the duration to achieve the desired timing for your animations.
Adjusting Easing Functions
Easing functions define how animations accelerate or decelerate over time. We'll demonstrate how to modify easing functions to create unique animation curves that match your project's style.
Combining Multiple Effects
For more complex text animations, combining multiple effects is essential. We'll guide you through the process of creating intricate animations by mixing different variants.
Adding Delay to Animations
Delays can create a more dynamic and synchronized feel in your animations. We'll teach you how to add delays to individual letters or words, enhancing the overall impact of your text animations.
Import Statements:
- We import the motion object from the "framer-motion" library, which is used to create animations.
- We import PropTypes for type checking.
- We import the varFadeInUp variant from a file named "variants."
- We import the Typography component from Material-UI for text rendering.
PropTypes:
- We define PropTypes for the TextAnimate component. It specifies the expected data types for the text, variants, and sx props.
TextAnimate Component Function:
- The TextAnimate component is a functional component that takes in text, variants, sx, and others as props.
Typography Component:
- Inside the TextAnimate component, we return a Typography component.
- We use Typography to control the text rendering with specific typography settings.
Typography Properties:
- We set the component property of Typography to motion.h1, which means our text will be wrapped in a motion.h1 element for animation purposes.
- We set various styles (sx) for the Typography component. These styles include typography settings such as "h1," as well as overflow and display styles. These styles are passed through the ...sx property.
- The ...other property allows for additional properties to be passed to the Typography component.
Text Splitting:
- Inside the Typography component, we split the text prop into individual letters using text.split(""). This allows us to animate each letter individually.
Mapping Over Letters:
- We map over the individual letters, creating a motion.span element for each letter.
- The key for each motion.span element is set to the index to help React efficiently update and manage these animation components.
Animation Variants:
- For each motion.span, we apply the variants property. If no specific variants are provided, it defaults to varFadeInUp.
varFadeInUp Variant:
- The varFadeInUp variant is not provided in the code you shared. However, this is where you would define the animation behavior, including initial and exit states, animation properties, and easing curves.
Practical Applications & Building Interactive Headers
Headers are the first thing users see, making them a perfect candidate for text animations. We'll provide real-world examples of how to create interactive and eye-catching headers for your web applications.
Animating Text on Button Clicks
Interactive elements like buttons can trigger animations. We'll show you how to animate text when a button is clicked, creating a seamless user experience.
Creating Attention-Grabbing Banners
Banners are often used for announcements or promotions. We'll demonstrate how to use text animations to make your banners stand out and capture your audience's attention.
Fine-Tuning Animation & Adjusting Animation Speed
Fine-tuning the animation speed is essential to ensure your animations feel just right. We'll provide tips and techniques for adjusting the animation speed to match your project's requirements.
Customizing Easing Curves
Easing curves can significantly impact the feel of your animations. We'll delve into customizing easing curves to create animations that perfectly match your project's design language.
Tweaking Initial and Exit States
Controlling the initial and exit states of your animations is crucial for achieving a polished look. We'll guide you through tweaking these states to ensure your text animations start and end seamlessly.
Real-World Examples & Testimonials and Reviews
Testimonials and reviews are often featured on websites. We'll showcase real-world examples of how text animations can be applied to make these sections more engaging and attention-grabbing.
Product Features Showcase
Product feature showcases are critical for marketing. We'll demonstrate how text animations can be used to highlight and explain product features in a visually appealing way.
Dynamic Content Loading
Dynamic content loading often benefits from animations. We'll show you how to use animations to enhance the loading of dynamic content and keep your users engaged.
Optimizing for Performance & Lazy Loading Animations
To ensure your application remains performant, you'll want to implement lazy loading for animations. We'll provide insights into how to load animations only when necessary, conserving resources.
Minimizing DOM Manipulation
Optimizing the number of DOM manipulations is essential for maintaining good performance. We'll explore techniques to minimize DOM updates while still achieving smooth text animations.
Reducing Animation Complexity
Complex animations can tax your application. We'll discuss strategies for reducing animation complexity to ensure your animations remain fluid and responsive.
Output:
Let us keep on learning
In conclusion, harnessing the power of React, Material-UI and Framer Motion for text animations opens up a world of creative possibilities for web designers and developers. The seamless integration of these technologies allows for the creation of visually striking and captivating text animations that can transform any website into an engaging and memorable user experience.
Stay updated for more blogs that delve into advanced topics of React, Material-UI and Framer Motion.