Implementing Authentication Security in a React App with Auth0
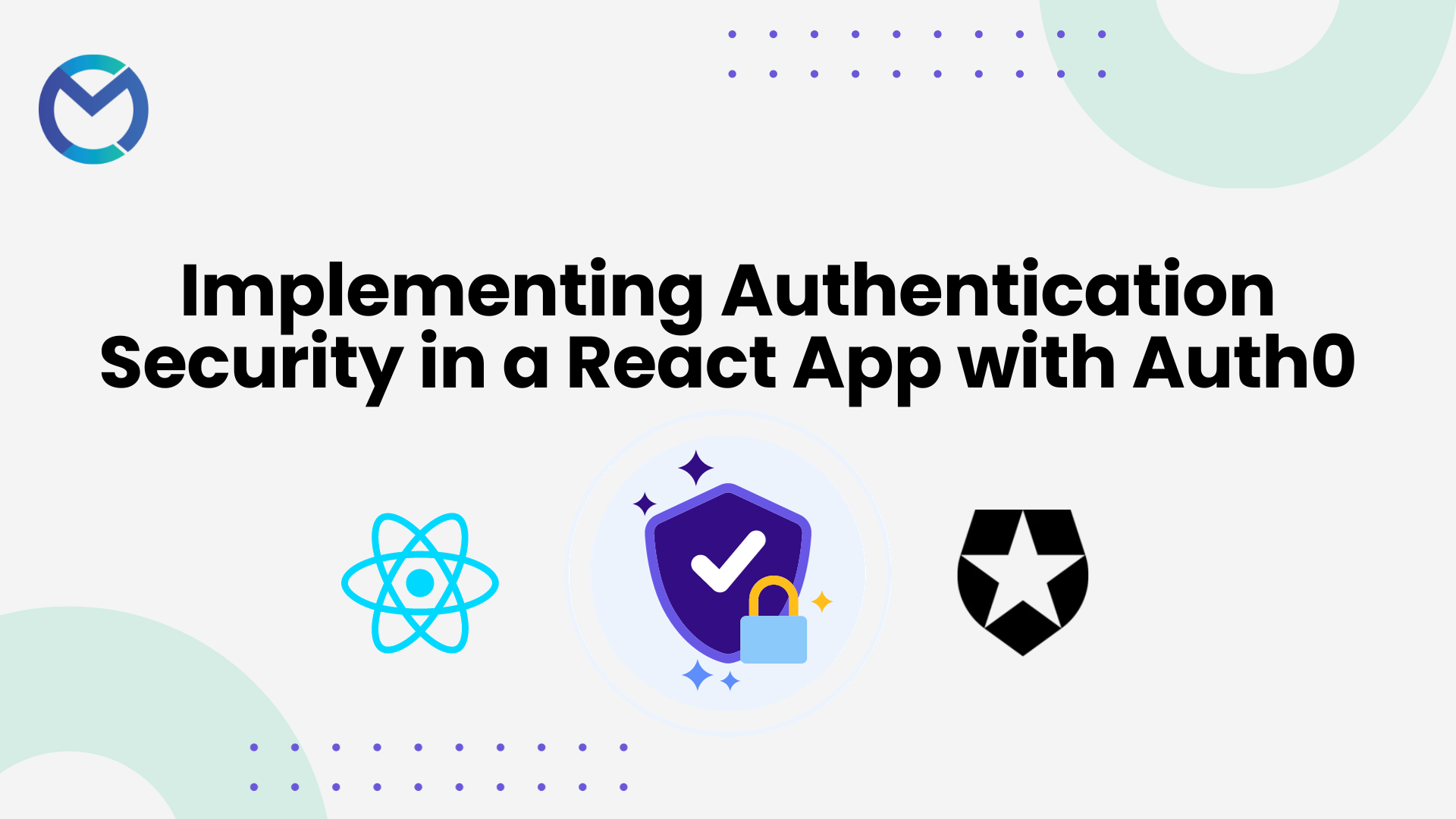
Authentication is a crucial aspect of web applications, ensuring that users' identities are verified and their data remains secure. In this tutorial, we'll explore how to implement authentication security in a React application using Auth0, a popular and robust authentication and authorization platform.
What is Auth0?
Auth0 is an identity and access management platform that provides developers with the tools to add robust authentication and authorization features to their applications. It simplifies the process of implementing secure user authentication, offering features like social logins, multi-factor authentication and user management.
Why Choose Auth0?
- Ease of Integration:
- Auth0 provides a seamless integration process, allowing developers to add authentication to their applications with minimal effort.
- It supports various identity providers, making it easy to implement social logins and other authentication methods.
- Security Features:
- Auth0 takes care of security best practices, including secure storage of user credentials and protection against common vulnerabilities.
- It offers features like multi-factor authentication, anomaly detection, and brute-force protection to enhance security.
- Scalability:
- Auth0 is designed to scale with your application. Whether you're building a small project or a large enterprise application, Auth0 can handle your authentication needs.
- User Management:
- Auth0 simplifies user management, allowing developers to focus on building features rather than dealing with the complexities of user registration, password recovery, and profile management.
How Auth0 Works
Auth0 follows a straightforward authentication flow:
- User Authentication:
- When a user tries to log in, Auth0 handles the authentication process. This can include traditional username/password authentication or social logins.
- Token Generation:
- Upon successful authentication, Auth0 generates tokens (e.g., JWTs) that can be used to securely identify the user.
- Token Verification:
- These tokens are sent with each request to the server, allowing the server to verify the user's identity without the need for constant reauthentication.
- User Management and Security Policies:
- Auth0 provides a dashboard for managing users, roles, and security policies. Developers can configure rules to implement custom authentication logic.
Setting Up Auth0
Before we dive into the React integration, let's set up Auth0:
- Create an Auth0 Account:
- Visit Auth0 and sign up for a free account.
- Create an Auth0 Application:
- Once logged in, navigate to the Auth0 Dashboard and create a new application.
- Configure the application settings, including the allowed callback URLs (e.g., http://localhost:3000 for development).
In this tutorial, we'll walk through the process of implementing authentication security in a React application using Auth0. The provided code will serve as the foundation for our application.
Prerequisites
Before we begin, make sure you have the following:
- Node.js and npm are installed on your machine.
- An Auth0 account for creating and managing authentication applications.
Step 1: Set Up Auth0
- Create an Auth0 Account:
- Visit Auth0 and sign up for a free account.
- Create an Auth0 Application:
- Once logged in, navigate to the Auth0 Dashboard and create a new application.
- Configure the application settings, including the allowed callback URLs (e.g., http://localhost:3000 for development).
Step 2: Create a React App
Let's create a new React app using Create React App.
npx create-react-app react-auth0-app
cd react-auth0-app
Step 3: Install Dependencies
Install the required dependencies, including the Auth0 SDK.
npm install @auth0/auth0-spa-js prop-types
Step 4: Replace the src/auth/AuthProvider.js file
Replace the content of src/auth/AuthProvider.js with the provided code.
import { createContext, useEffect, useReducer } from 'react';
import PropTypes from 'prop-types';
import { Auth0Client } from '@auth0/auth0-spa-js';
import { auth0Config } from '../config';
let auth0Client = null;
const initialState = {
isAuthenticated: false,
isInitialized: false,
user: null
};
const handlers = {
INITIALIZE: (state, action) => {
const { isAuthenticated, user } = action.payload;
return { ...state, isAuthenticated, isInitialized: true, user };
},
LOGIN: (state, action) => {
const { user } = action.payload;
return { ...state, isAuthenticated: true, user };
},
LOGOUT: (state) => ({
...state,
isAuthenticated: false,
user: null
})
};
const reducer = (state, action) => (handlers[action.type] ? handlers[action.type](state, action) : state);
const AuthContext = createContext({
...initialState,
method: 'auth0',
login: () => Promise.resolve(),
logout: () => Promise.resolve()
});
AuthProvider.propTypes = {
children: PropTypes.node
};
function AuthProvider({ children }) {
const [state, dispatch] = useReducer(reducer, initialState);
useEffect(() => {
const initialize = async () => {
try {
auth0Client = new Auth0Client({
client_id: auth0Config.clientId,
domain: auth0Config.domain,
redirect_uri: window.location.origin
});
await auth0Client.checkSession();
const isAuthenticated = await auth0Client.isAuthenticated();
if (isAuthenticated) {
const user = await auth0Client.getUser();
dispatch({
type: 'INITIALIZE',
payload: { isAuthenticated, user }
});
} else {
dispatch({
type: 'INITIALIZE',
payload: { isAuthenticated, user: null }
});
}
} catch (err) {
console.error(err);
dispatch({
type: 'INITIALIZE',
payload: { isAuthenticated: false, user: null }
});
}
};
initialize();
}, []);
const login = async () => {
await auth0Client.loginWithPopup();
const isAuthenticated = await auth0Client.isAuthenticated();
if (isAuthenticated) {
const user = await auth0Client.getUser();
dispatch({ type: 'LOGIN', payload: { user } });
}
};
const logout = () => {
auth0Client.logout();
dispatch({ type: 'LOGOUT' });
};
const resetPassword = () => { };
return (
<AuthContext.Provider
value={{
...state,
method: 'auth0',
user: {
id: state?.user?.sub,
photoURL: state?.user?.picture,
email: state?.user?.email,
displayName: 'Jaydon Frankie',
role: 'admin'
},
login,
logout,
resetPassword
}}
>
{children}
</AuthContext.Provider>
);
}
export { AuthContext, AuthProvider };
Step 5: Configure Auth0
Open src/config.js and replace the configuration with your Auth0 application details.
export const auth0Config = {
domain: 'your-auth0-domain',
clientId: 'your-auth0-client-id'
};
Step 6: Integrate Auth0 into Components
Now, you can use the AuthContext in your components to handle authentication. For example, in src/components/YourComponent.js:
import React, { useContext } from 'react';
import { AuthContext } from '../auth/AuthProvider';
const YourComponent = () => {
const { isAuthenticated, login, logout, user } = useContext(AuthContext);
return (
<div>
{isAuthenticated ? (
<>
<p>Welcome, {user.displayName}!</p>
<button onClick={logout}>Logout</button>
</>
) : (
<button onClick={login}>Login</button>
)}
</div>
);
};
export default YourComponent;
Step 7: Adding a Login Form
To allow users to log in to your application, you can create a login form component. Below is an example of a basic login form using React and the AuthContext.
Create a new file, LoginForm.js, and add the following code:
import React, { useContext, useState } from 'react';
import { AuthContext } from '../auth/AuthProvider';
const LoginForm = () => {
const { login } = useContext(AuthContext);
const [email, setEmail] = useState('');
const [password, setPassword] = useState('');
const handleLogin = async (e) => {
e.preventDefault();
await login();
};
return (
<div>
<h2>Login</h2>
<form onSubmit={handleLogin}>
<label>
Email:
<input
type="email"
value={email}
onChange={(e) => setEmail(e.target.value)}
required
/>
</label>
<br />
<label>
Password:
<input
type="password"
value={password}
onChange={(e) => setPassword(e.target.value)}
required
/>
</label>
<br />
<button type="submit">Login</button>
</form>
</div>
);
};
export default LoginForm;
App.js
import React from 'react';
import { AuthProvider } from './auth/AuthProvider';
import LoginForm from './components/LoginForm';
const App = () => {
return (
<AuthProvider>
<div>
<LoginForm />
</div>
</AuthProvider>
);
};
export default App;
Step 8: Start the React App
Start your React app and see Auth0 authentication in action.
npm start
Conclusion
By following this tutorial, you've not only implemented authentication security in your React application using Auth0 but have also added a functional login form for a seamless user experience.