Mastering Theming in Chakra UI: Elevate Your Design Game
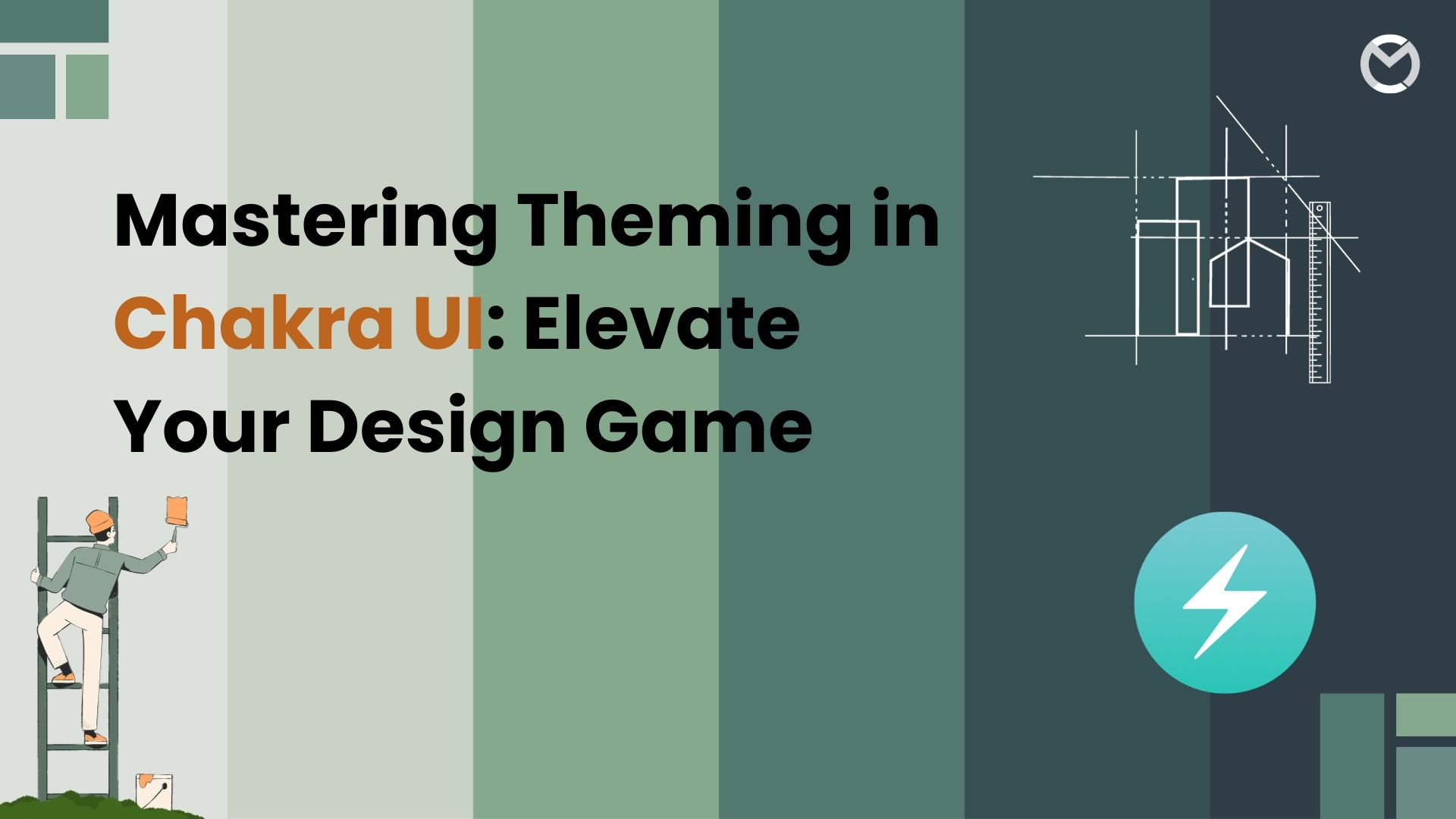
When it comes to building web applications, the look and feel of your project play a pivotal role in attracting and engaging users. Chakra UI, a popular React component library, offers powerful theming capabilities that can help you create beautiful and consistent user interfaces effortlessly. In this blog post, we'll guide you through the process of mastering advanced theming in Chakra UI by building a stunning demo application.
Prerequisites
Before we dive into building our demo application, make sure you have the following prerequisites in place:
- Node.js and npm installed on your system.
- Basic knowledge of React.
Step 1: Setting Up a React Application
Our journey begins by creating a new React application using Create React App. Open your terminal and run the following commands:
npx create-react-app chakra-ui-demo
cd chakra-ui-demo
npm i @chakra-ui/react @emotion/react @emotion/styled framer-motion
This will set up a new React application and install the necessary dependencies, including Chakra UI.
Step 2: Creating a ChakraProvider
To enable Chakra UI theming in our application, we need to wrap the entire app in the ChakraProvider
component. Open src/index.js
and modify it as follows:
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
import { ChakraProvider } from '@chakra-ui/react';
ReactDOM.render(
<ChakraProvider>
<React.StrictMode>
<App />
</React.StrictMode>
</ChakraProvider>,
document.getElementById('root')
);
This sets up the Chakra UI provider for our app.
Step 3: Defining a Custom Theme
Chakra UI theming begins with defining a custom theme that includes customizations for colors, fonts, spacing, and more. Create a new file src/theme.js
and define your custom theme:
const customTheme = {
colors: {
primary: '#3498db',
secondary: '#2ecc71',
background: '#f0f0f0',
text: '#333',
},
fonts: {
heading: 'Roboto, sans-serif',
body: 'Arial, sans-serif',
},
fontSizes: {
small: '14px',
medium: '16px',
large: '18px',
},
fontWeights: {
normal: 400,
bold: 700,
},
spacing: {
small: '8px',
medium: '16px',
large: '24px',
},
breakpoints: {
sm: '480px',
md: '768px',
lg: '1024px',
xl: '1280px',
},
};
export default customTheme;
In this example, we've customized colors and fonts. You can add more customizations as needed.
Step 4: Applying the Custom Theme
Now, let's apply the custom theme to our application. In src/index.js
, import the custom theme and apply it using the extendTheme
function:
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
import { ChakraProvider, extendTheme } from '@chakra-ui/react';
import customTheme from './theme';
const theme = extendTheme(customTheme);
ReactDOM.render(
<ChakraProvider theme={theme}>
<React.StrictMode>
<App />
</React.StrictMode>
</ChakraProvider>,
document.getElementById('root')
);
This code configures Chakra UI with our custom theme.
Step 5: Creating a Sample Component
For our demo, we'll create a simple component to showcase theming. In src/ThemedComponent.js
, create the component:
import React from 'react';
import { Box, Text, Button } from '@chakra-ui/react';
function ThemedComponent() {
return (
<Box bg="primary.500" p={4}>
<Text fontFamily="heading" color="white">
This is a themed component.
</Text>
<Button variant="primary" mt={4}>
Themed Button
</Button>
</Box>
);
}
export default ThemedComponent;
In this component, we've used our custom theme's colors, fonts, and a customized button variant.
Step 6: Using the Component in App.js
In src/App.js
, include our ThemedComponent
within your app:
import React from 'react';
import './App.css';
import ThemedComponent from './ThemedComponent';
function App() {
return (
<div className="App">
<ThemedComponent />
</div>
);
}
export default App;
Step 7: Starting the Development Server
To see your stunning demo application in action, run the development server with the following command:
npm start
Conclusion: Join the Creative Journey!
We've explored the art of creating custom themes, from defining color palettes to customizing typography. Your design skills are now armed with the knowledge and tools to create visually captivating web applications.
But our journey doesn't end here. I've used Bun.js to build the demo application which just a way to explain the theming and define a custom theme. I've made the code available in a repository, and I would like you to take it to the next level.
I haven't added an extensive amount of code to the project. Instead, I've concentrated on explaining the intricate details of theming in Chakra UI, leaving room for your creativity to flourish.
Feel free to clone the repository, experiment with the theme, and create exciting UI components. Change colors, fonts, and styles to match your creative vision. Whether it's a personal project, a portfolio piece, or an exploration of new design ideas, the code is yours to innovate upon.
Your Contributions Matter! If you decide to make exciting changes, don't hesitate to send a pull request. I'd love to see how you've taken the project to new heights. Collaborative design can lead to exceptional results, and I look forward to witnessing your contributions.
Remember, in the world of web development and design, every change you make, no matter how small, contributes to a vibrant and ever-evolving community. So go ahead, clone the repository, and let your creativity flow.
If you want to know about bun js, you can read my previous blog : https://blogs.mulecraft.in/bun-js-a-bright-future-for-javascript/
Thank you for joining me on this creative journey, and I can't wait to see the exciting changes you'll bring to the project!
Happy theming and designing!