React Roadmap Series 1 - Basic HTML, CSS, JS and ES6
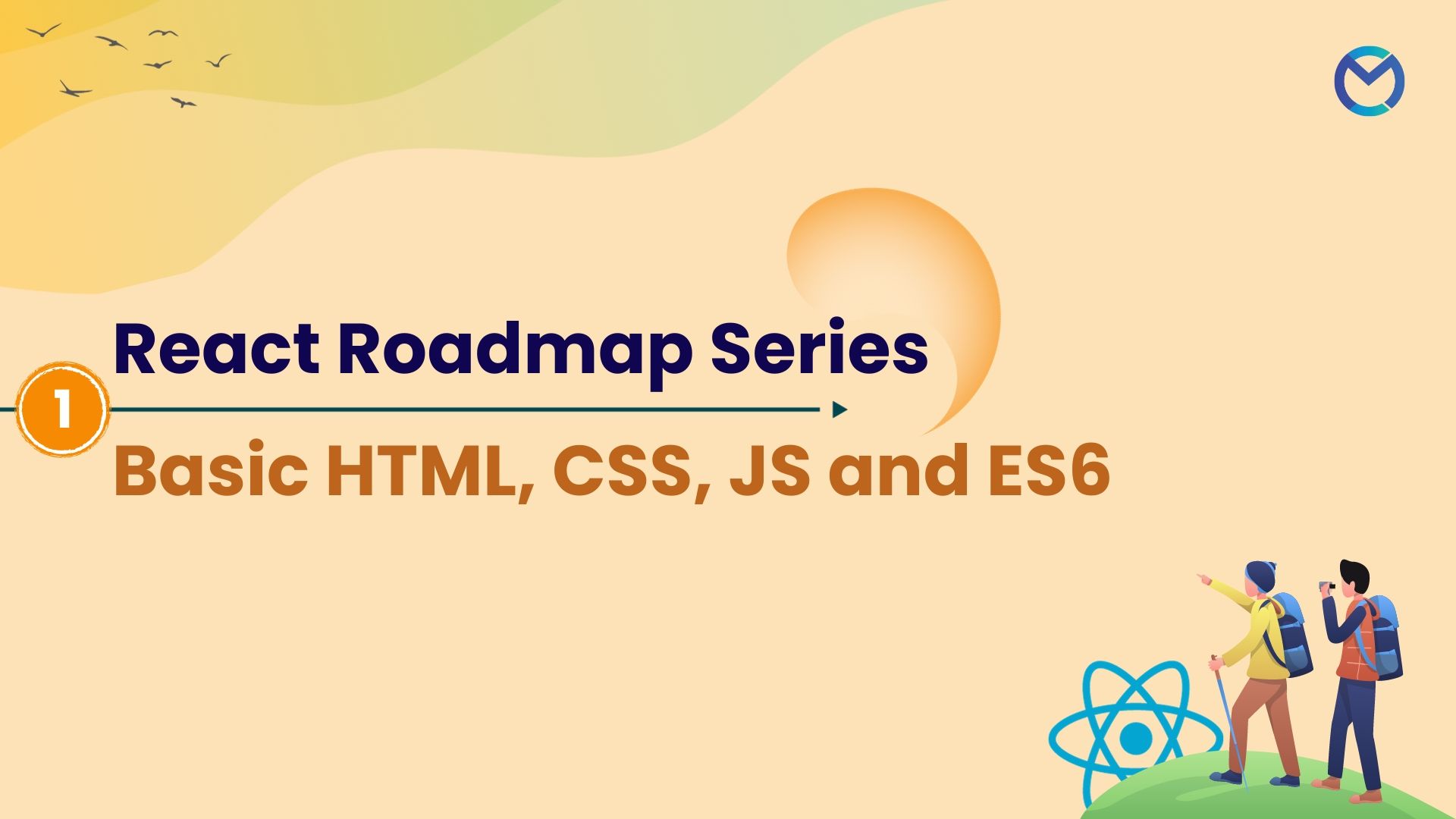
Choosing a frontend library or framework is a decision that depends on various factors, including project requirements, team expertise and personal preferences. Here are some reasons why one might choose React over other popular frontend libraries:
- Component-Based Architecture
- Virtual DOM and Performance
- One-Way Data Binding
- JSX Syntax
- Large Ecosystem and Community
- Flexibility with State Management
- Backed by Facebook
- React Native for Cross-Platform Development
- Tooling and DevTools
- Adoption by Major Companies
Blog Series
In the ever-evolving landscape of frontend technologies, mastering React stands as a transformative journey that opens doors to building interactive, efficient and scalable user interfaces. If you are stepping into the realm of web development, then this blog series might be your little guide in unraveling the secrets of React JS.
In order to learn React, we need to know the basics of HTML, CSS and JavaScript. If you are totally new to them, kindly spend some time to learn its basics as we won't be diving deep into it.
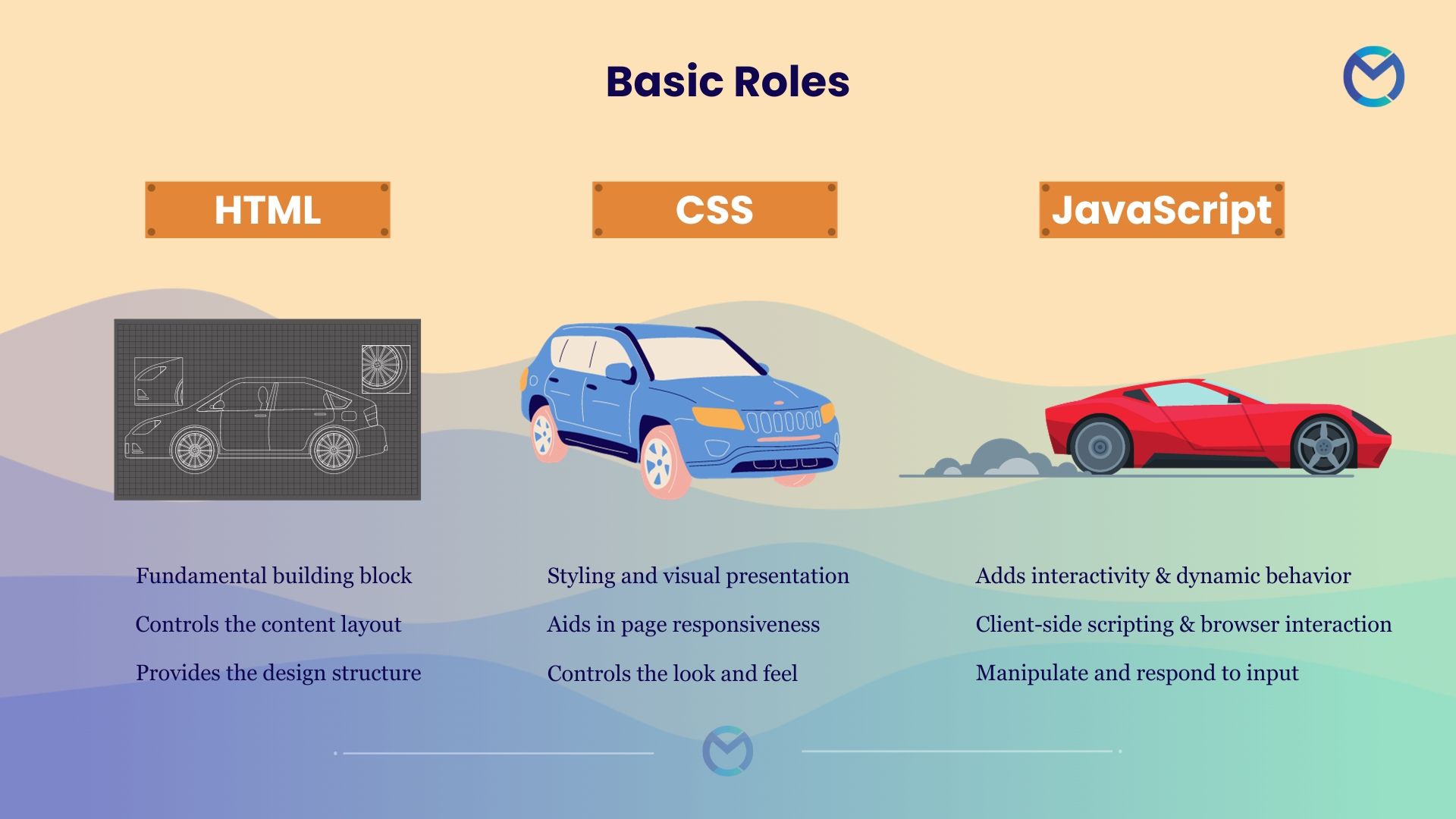
Hyper Text Markup Language (HTML)
HTML forms the backbone of web development. It provides the structure and semantics needed to create web pages.
HTML is composed of elements and attributes. Elements are the building blocks of HTML documents and represent the structure of the content. An HTML element usually consists of a start tag, content, and an end tag. For example:
<p>MuleCraft is your ideal tech companion.</p>
In this example, <p>
is the start tag, MuleCraft is your ideal tech companion.
is the content and </p>
is the end tag.
Attributes provide additional information about HTML elements and are always included in the opening tag. They are typically used to modify or extend the behaviour of an element. For instance:
<a href="https://www.mulecraft.in">Visit MuleCraft</a>
In this <a>
(anchor) element, href
is an attribute that specifies the hyperlink reference. Attributes are crucial for defining the behaviour, appearance and functionality of HTML elements within a web page.
Checkout the list of some of the most commonly used HTML elements.
<html>
: Defines the root of an HTML document.<head>
: Contains meta-information about the HTML document.<title>
: Sets the title of the HTML document (appears in the browser tab).<body>
: Contains the content of the HTML document.<h1> to <h6>
: Define heading levels (1 being the highest and 6 the lowest).<p>
: Represents a paragraph of text.<a>
: Creates hyperlinks.<img>
: Embeds images.<ul>
: Defines an unordered (bulleted) list.<ol>
: Defines an ordered (numbered) list.<li>
: Represents a list item within<ul>
or<ol>
.<div>
: Defines a division or a container for other elements.<span>
: Defines an inline container for text or other inline elements.<input>
: Creates an input field, commonly used in forms.<form>
: Defines an HTML form for user input.<button>
: Represents a clickable button.<table>
: Creates an HTML table.<tr>
: Defines a table row.<td>
: Represents a table cell.
HTML5 has a defined set of around 140 elements. These elements cover a wide range of functionalities, from defining the basic structure of a document to embedding multimedia, creating forms and enabling interactivity.
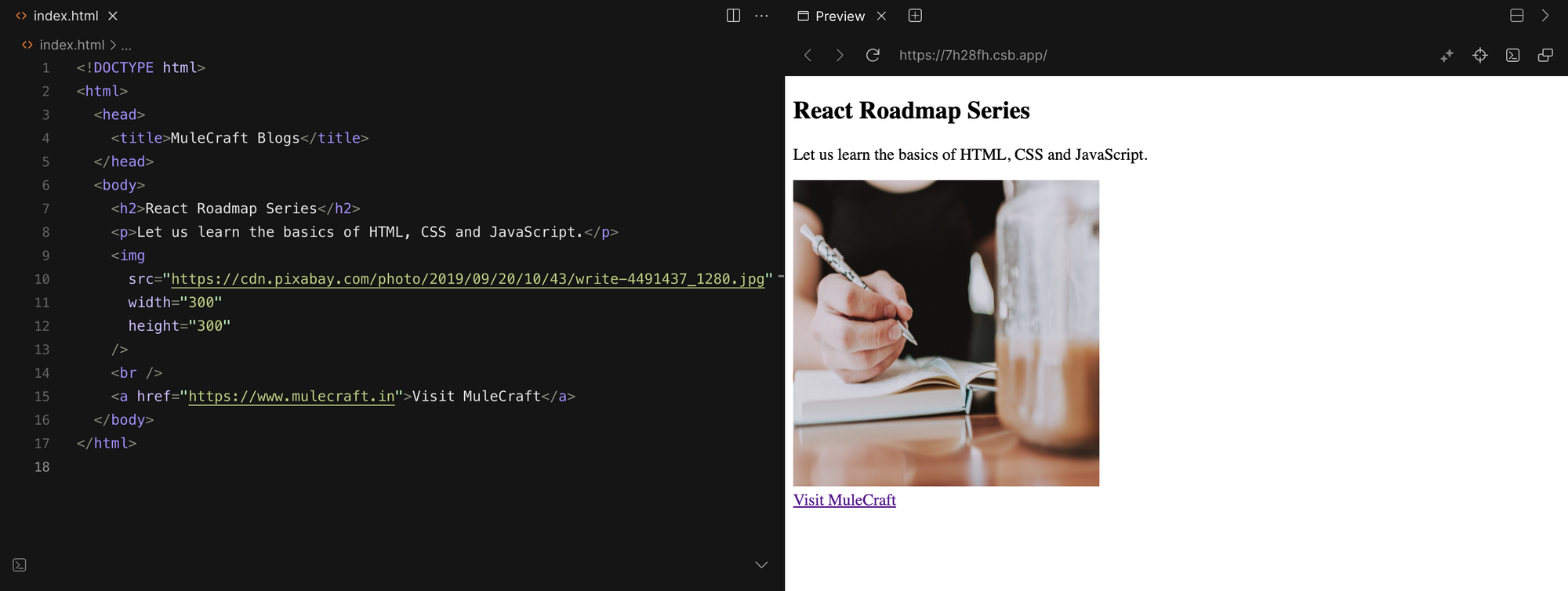
Cascading Style Sheets (CSS)
CSS is a style sheet language used for describing the presentation and layout of a document written in HTML or XML. CSS enhances the visual presentation of web pages by defining how elements should be styled, positioned and displayed on the screen.
Types
- Inline CSS
Applied directly to an HTML element using the style
attribute.
<p style="color: blue;">This is a blue paragraph.</p>
- Internal or Embedded CSS
Defined within the HTML document, typically in the head section using the <style>
tag.
<head>
<style>
p {
color: red;
}
</style>
</head>
- External CSS
Stored in a separate CSS file and linked to the HTML document. This promotes better code organization and reusability.
<head>
<link rel="stylesheet" type="text/css" href="styles.css">
</head>
Components of CSS
- Selectors
Selectors target HTML elements to apply styling rules. They can be based on element types, classes, IDs, attributes, or combinations.
- Properties
Properties define the visual characteristics of the selected elements, such as color, font size, margin, padding, etc.
- Values
Values are assigned to properties and determine the specific style that will be applied. For example, the value for the color property could be "red" or "#00ff00" (hex code).
- Declaration
A declaration is a combination of a property and its value. Multiple declarations are grouped together to form a CSS rule.
/* styles.css */
p {
color: blue;
font-size: 16px;
margin-top: 10px;
}
In this example, the CSS rule targets <p>
elements and sets their color to blue, font size to 16 pixels and top margin to 10 pixels.
CSS allows developers to separate the structure (HTML) from the presentation (CSS) and facilitates the creation of visually appealing and responsive web designs.
JavaScript (JS)
JavaScript is a versatile and widely-used programming language that primarily runs in web browsers. It enables developers to create dynamic and interactive content on websites, enhancing user experience by manipulating the Document Object Model (DOM) and responding to user actions.
DOM represents the structure of a document as a tree of objects where each node in the tree corresponds to a part of the document, such as elements, attributes and text.
Here's a simplified example of HTML and its corresponding DOM representation:
<!– HTML Document -->
<!DOCTYPE html>
<html>
<head>
<title>Example</title>
</head>
<body>
<h1>Hello, World!</h1>
<p>This is a simple example.</p>
</body>
</html>
Corresponding DOM Tree:
- Document
- html
- head
- title
- "Example"
- body
- h1
- "Hello, World!"
- p
- "This is a simple example."
In this example, the HTML document is represented as a tree structure in the DOM, with nodes corresponding to elements and their content. Developers can use JavaScript to interact with this DOM tree, making it a central part of dynamic web development.
Types of JavaScript
- Client-Side JavaScript
Executed on the user's browser, enabling dynamic changes to web pages without requiring a server round-trip. Commonly used for form validation, animations and handling user interactions.
- Server-Side JavaScript
Used on the server side, allowing developers to write server applications. Node.js is a popular runtime that enables server-side JavaScript development.
- Frameworks and Libraries
JavaScript has various frameworks and libraries that simplify and accelerate the development process. Examples include React, Angular, Vue.js (frameworks) and jQuery (library).
Components of JS
- Variables: Used to store and manipulate data.
- Data Types: Include primitive types (e.g., number, string, boolean) and complex types (e.g., object, array).
- Operators: Used for performing operations on variables and values.
- Control Structures: Include if statements, loops and switch statements for controlling the flow of a program.
- Functions: Blocks of reusable code that can be invoked with specific inputs (arguments).
- Objects: Key-value pairs that allow the organization and manipulation of data.
- Events: Enable the execution of code in response to user actions or other events.
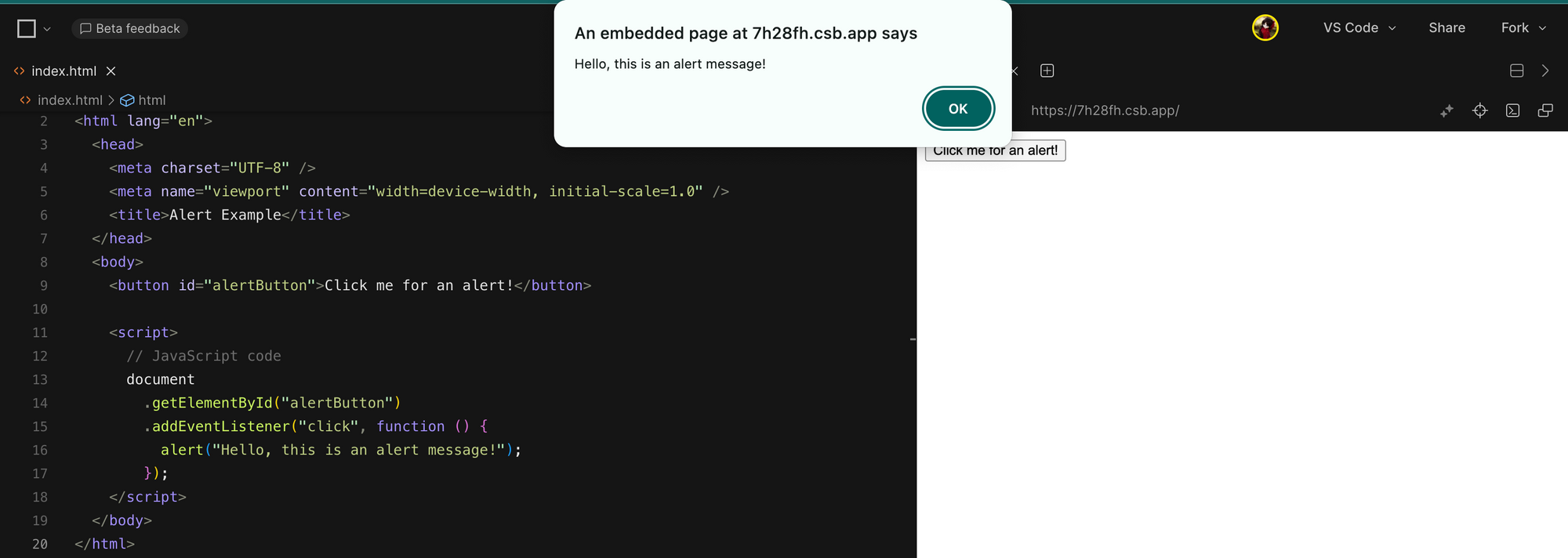
- There's a button with the id "alertButton".
- The JavaScript code uses
addEventListener
to attach a click event to the button. - When the button is clicked, the event handler function is triggered, which displays an alert message saying "Hello, this is an alert message!"
You can save this code in an HTML file and open it in a web browser to see the alert in action when the button is clicked.
The<script>
tag in HTML is used to embed or reference JavaScript code within an HTML document. It allows developers to include client-side scripts directly in the HTML file or link to external JavaScript files. The<script>
element can be placed in the<head>
or<body>
section of an HTML document.
ECMAScript 2015 (ES6)
ES6 is the scripting language specification that JavaScript is based on. It's important to note that ES6 is a version of JavaScript—it's not a separate language but an evolution of the JavaScript language itself. JavaScript engines in modern web browsers implement the ECMAScript specifications, including ES6 and subsequent versions.
ES6 introduced new syntax, features and improvements to make JavaScript more expressive, readable and powerful. It's like a set of new vocabulary and grammar rules added to an existing language, for the purpose of enhancement.
JavaScript (Pre-ES6)
// Traditional function
function add(a, b) {
return a + b;
}
JavaScript (ES6)
// Arrow function introduced in ES6
const add = (a, b) => a + b;
1. let and const Declarations
let
andconst
are block-scoped declarations for variables.let
allows reassignment, whileconst
is used for variables that should not be reassigned.
let count = 10;
count = 20; // Valid
const pi = 3.14;
pi = 3.14159; // Invalid
2. Arrow Functions
- Arrow functions provide a more concise syntax for writing functions.
const add = (a, b) => a + b;
3. Template Literals
- Template literals allow embedding expressions inside string literals, making string interpolation more readable.
const name = 'Shanmathy';
const greeting = `Hello, ${name}`;
4. De-structuring Assignment
- De-structuring enables extracting values from arrays or objects and assigning them to variables in a concise manner.
// Array de-structuring
const [first, second] = [1, 2];
// Object de-structuring
const person = { name: 'User', age: 20 };
const { name, age } = person;
5. Spread and Rest Operators
- The spread operator (
...
) is used to spread elements of an iterable (like an array) into another. The rest operator is used to collect the remaining elements into a single variable.
// Spread operator
const array1 = [1, 2, 3];
const array2 = [...array1, 4, 5];
// Rest operator
const sum = (first, second, ...rest) => first + second + rest.length;
Here, the sum arrow function takes three parameters: first
, second
and ...rest
. The first two are regular parameters, and ...rest
is a rest parameter. The rest parameter allows you to represent an indefinite number of arguments as an array. In this example, any additional parameters passed to the function beyond the first two (first and second) will be collected into the rest
array, making it flexible and handy for cases where you don't know how many arguments will be passed.
6. Classes
- ES6 introduced a more concise syntax for defining classes and working with inheritance.
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
greet() {
console.log(`Hello, my name is ${this.name}.`);
}
}
const user = new Person('MuleDev', 25);
user.greet();
7. Promises
- Promises provide a cleaner way to work with asynchronous code compared to callbacks.
const fetchData = () => {
return new Promise((resolve, reject) => {
// Asynchronous operation
if (/* operation successful */) {
resolve('Data fetched successfully');
} else {
reject('Error fetching data');
}
});
};
fetchData()
.then(data => console.log(data))
.catch(error => console.error(error));
8. Modules
- ES6 introduced a module system, allowing developers to organize code into separate files with imports and exports.
// module.js
export const pi = 3.14;
// main.js
import { pi } from './module';
These are just a few highlights of the features introduced in ES6.
Next stop on the roadmap - React Basics
We've touched upon the fundamentals that lay the foundation for building dynamic and interactive user interfaces. The next blog will deliver a comprehensive understanding of React basics, unraveling its core principles and guiding you through practical examples.
Subscribe and stay tuned !