React Roadmap Series 4 - State Management
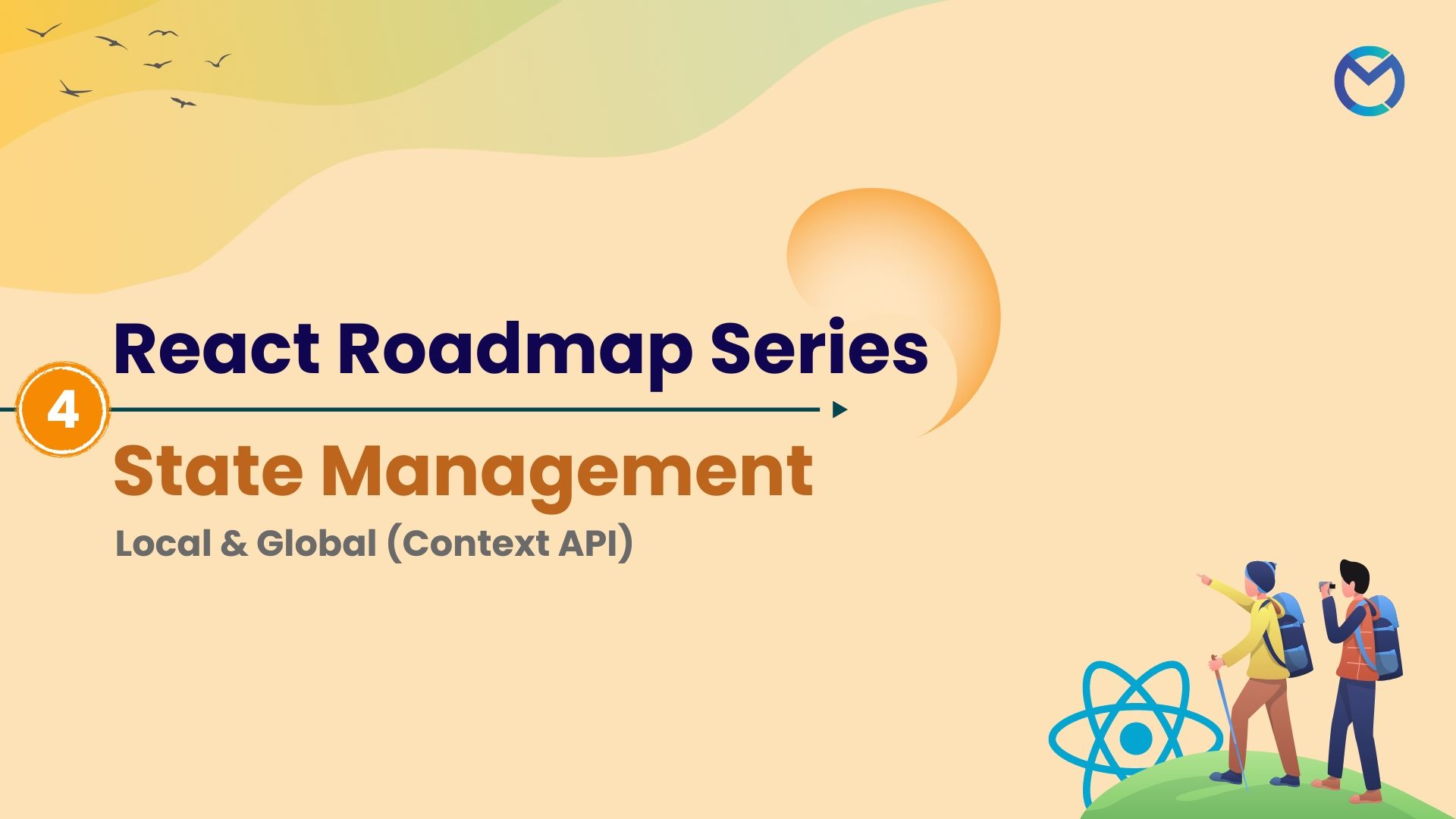
State management lies at the heart of every React application, determining how data is stored, accessed and modified throughout the lifecycle of components. Understanding state management is crucial for building robust and dynamic user interfaces.
In this blog, we'll explore the fundamentals of state management in React, demystify key concepts and understanding it better with practical examples, to ease our React journey.
Types
Local state management
Global state management
Local state management
Local state management in React involves managing state within a component using React's built-in state management system. This allows components to manage their own state without needing to rely on external libraries or global state management solutions. Here's how you can implement local state management in a React component:
Using Class Components
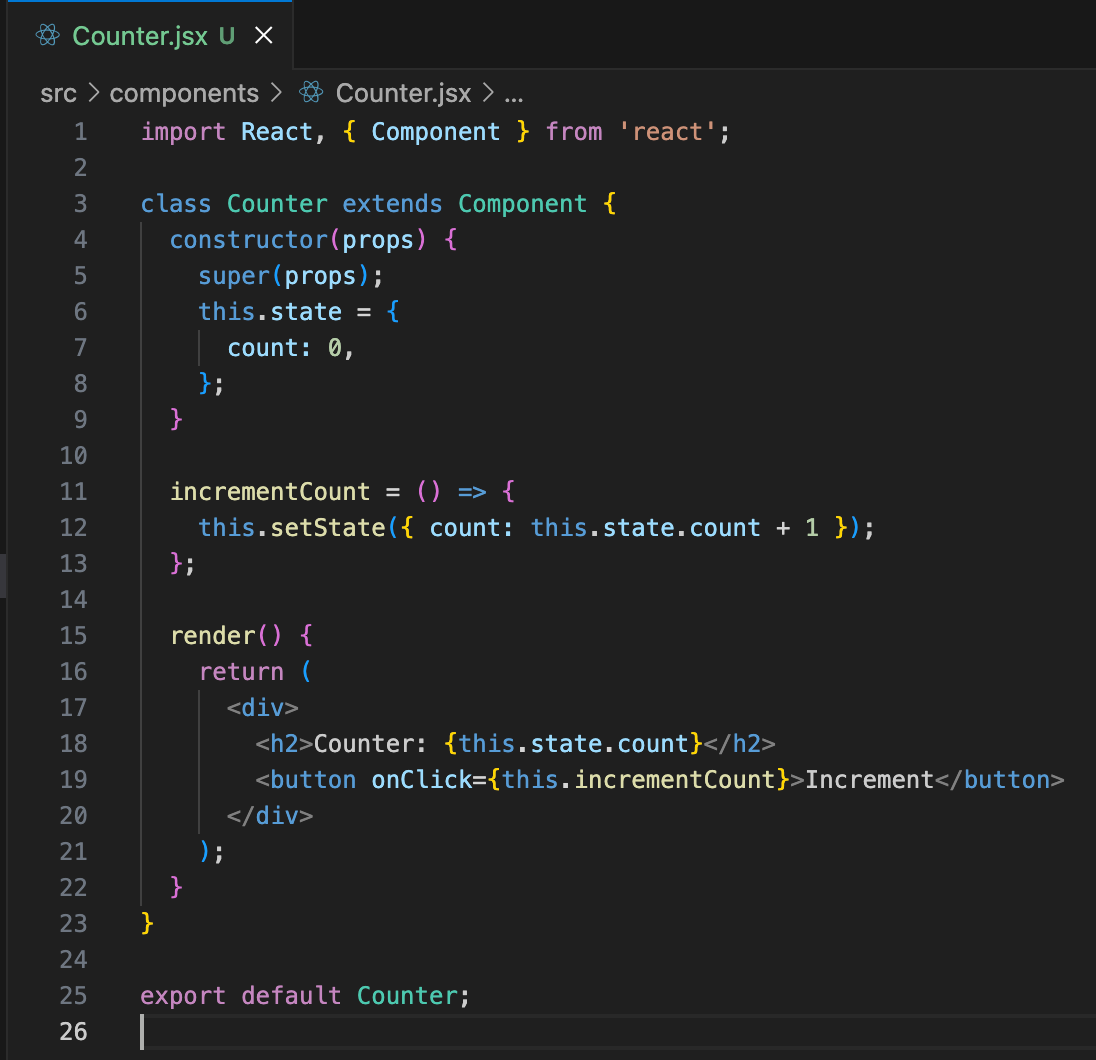
In this example, the Counter
component maintains its own local state using the state
property provided by React's class component. The count
state is initialized to 0 in the component's constructor, and the incrementCount
method is used to update the count state when the button is clicked.
Using Functional Components With Hooks
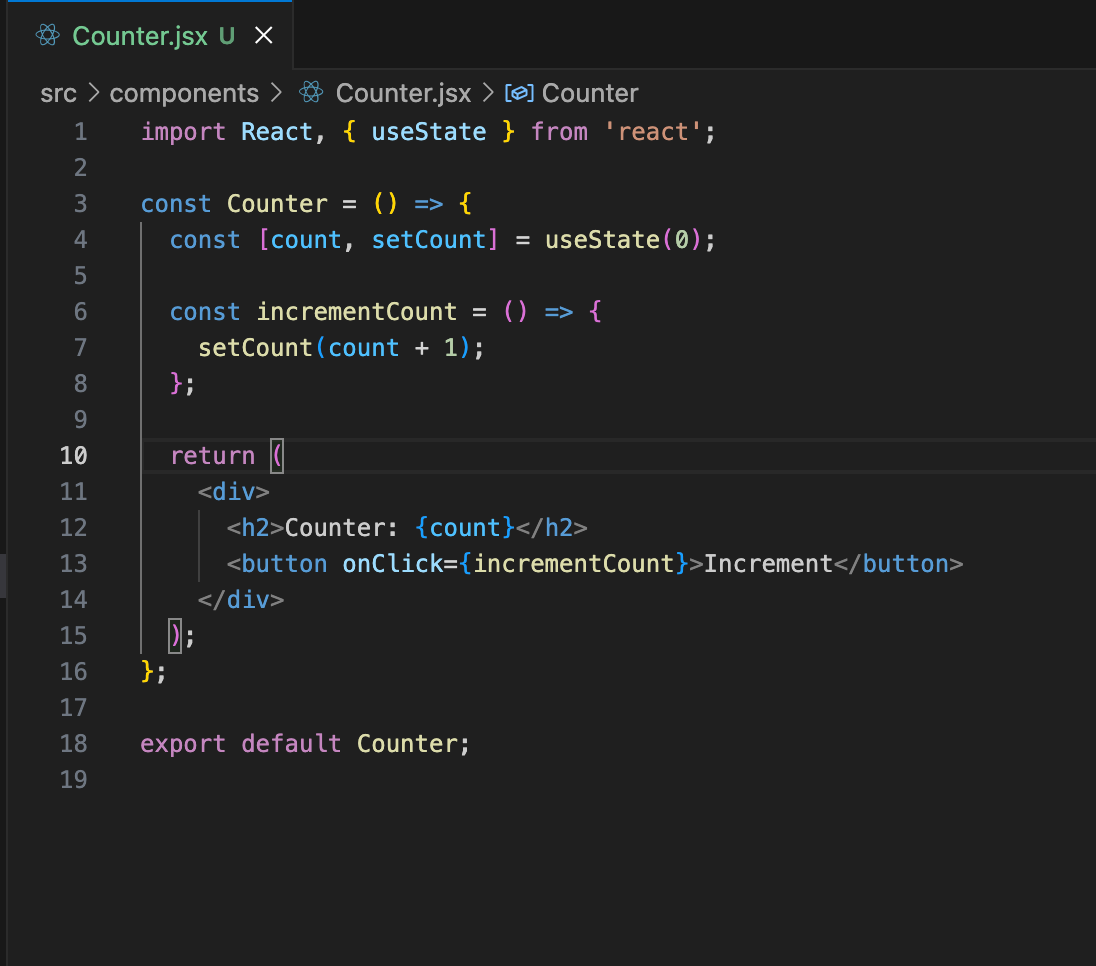
In this example, the Counter
component is implemented as a functional component using React hooks (useState
). The count
state and the setCount
function for updating the state are obtained by calling the useState
hook with an initial value of 0. The incrementCount
function updates the count state when the button is clicked.
Considerations
- Local state management is suitable for managing component-specific state that doesn't need to be shared with other components.
- State updates using
setState
(in class components) or state setter functions (in functional components with hooks) are asynchronous, so you should avoid relying on the current state value within the update function. - Use local state management for simple state needs within individual components, but consider using global state management solutions for complex state management across multiple components.
By implementing local state management in React components, you can efficiently manage component-specific state and create interactive user interfaces with ease.
Global State Management
Global state management involves managing state that needs to be accessible across different components in your React application. Instead of passing props down through multiple levels of the component tree, global state allows you to access and update state from any component within your application.
To achieve global state management, we can opt for one of the following:
Context API
Redux
React Query
Let us discuss in detail about Context API in this blog.
Context API
The Context API is a built-in feature of React that allows you to create global state that can be accessed by any component in your application. Here's how you can use the Context API for global state management:
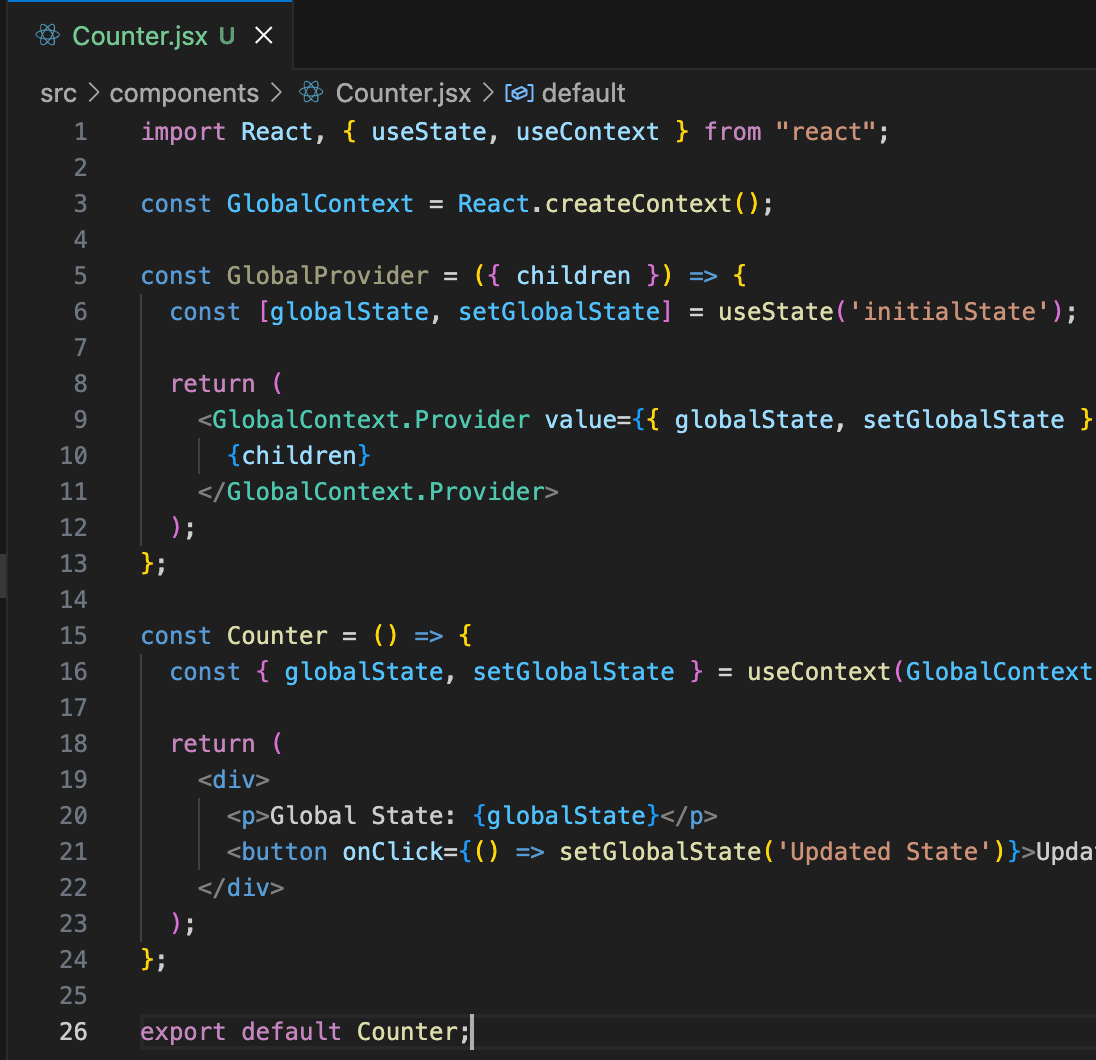
const GlobalContext = React.createContext();
Here, we're creating a new context using React.createContext()
. This context will be used to provide global state to components within our application.
const GlobalProvider = ({ children }) => {
const [globalState, setGlobalState] = useState('initialState');
return (
<GlobalContext.Provider value={{ globalState, setGlobalState }}>
{children}
</GlobalContext.Provider>
);
};
We define a GlobalProvider
component that takes children
as its prop. Inside this component, we use the useState
hook to create a piece of state called globalState
, initialized with some initial value (initialState
). We then use GlobalContext.Provider
to wrap the children
components, passing down the globalState
and setGlobalState
as the value of the provider.
const { globalState, setGlobalState } = useContext(GlobalContext);
In any component where we want to access the global state, we use the useContext
hook to consume the GlobalContext
. This allows us to access globalState
and setGlobalState
. We can then use globalState
to display the current state and setGlobalState
to update the state when needed.
So, in summary, the GlobalProvider
component serves as the provider for the global state, and components can access and update this global state using the useContext
hook.
You can export the GlobalProvider and wrap it around your target component, so that it can access the global state provided by the GlobalContext
.
<GlobalProvider>
<div>
<MyComponent />
</div>
</GlobalProvider>
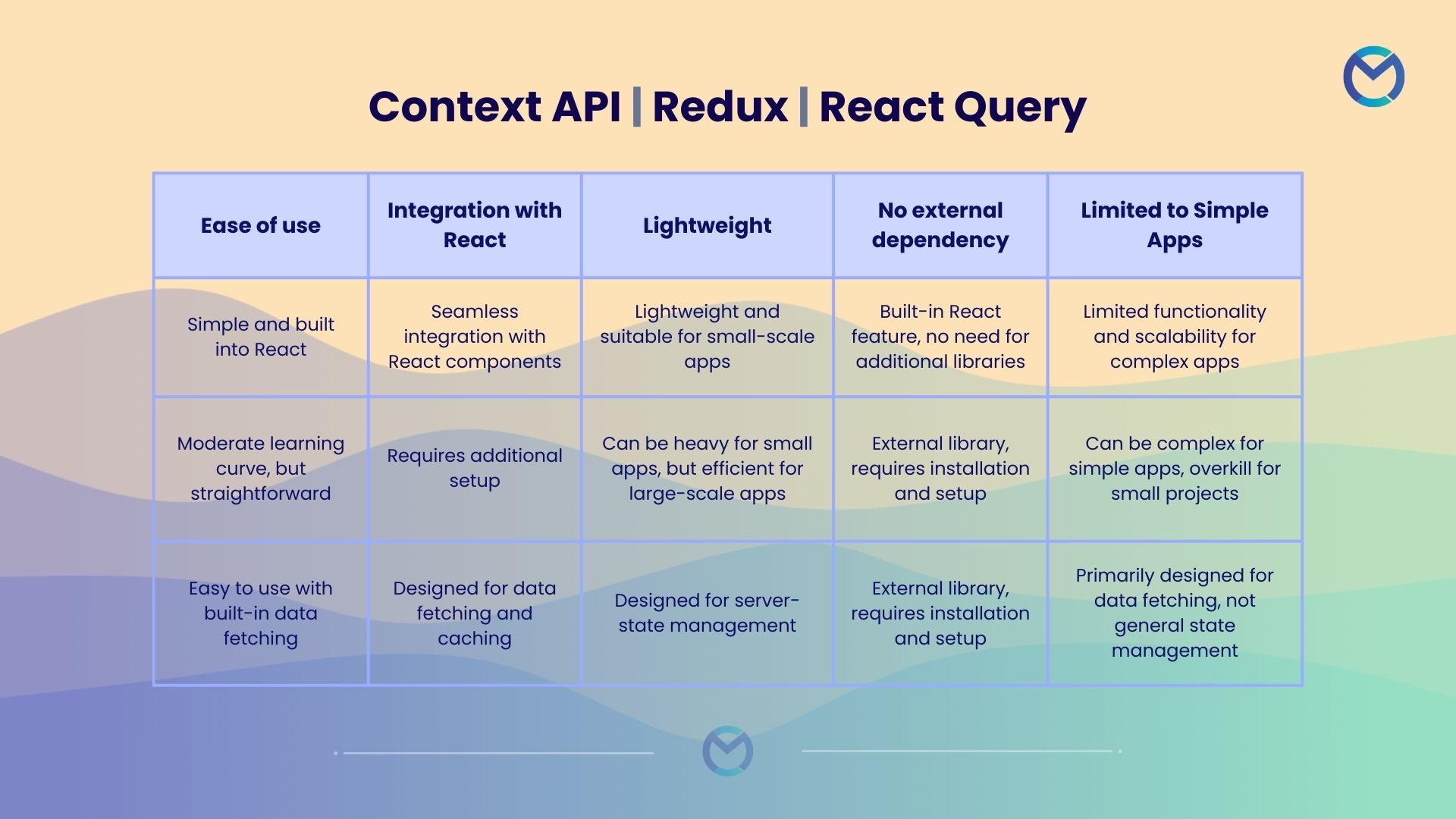
Conclusion
In conclusion, state management is a crucial aspect of building React applications, and understanding both local and global state management is essential for creating robust and scalable applications. Throughout this blog, we have explored the Context API for managing both local and global state in React applications. We've learned how to use context providers and consumers to share state across components efficiently.
By mastering these techniques, developers can build more maintainable and organized codebases, improving the overall development experience and enhancing the user experience. However, it's important to note that the Context API has its limitations, especially for larger and more complex applications.
In the next blog, we will delve deeper into advanced state management techniques using Redux and React Query. These libraries offer powerful features for managing state in React applications, including centralized state management, time-travel debugging, and efficient data fetching and caching. Stay tuned for an in-depth exploration of these tools and their applications in building modern React applications.