React Roadmap Series 2 - Understanding React Basics
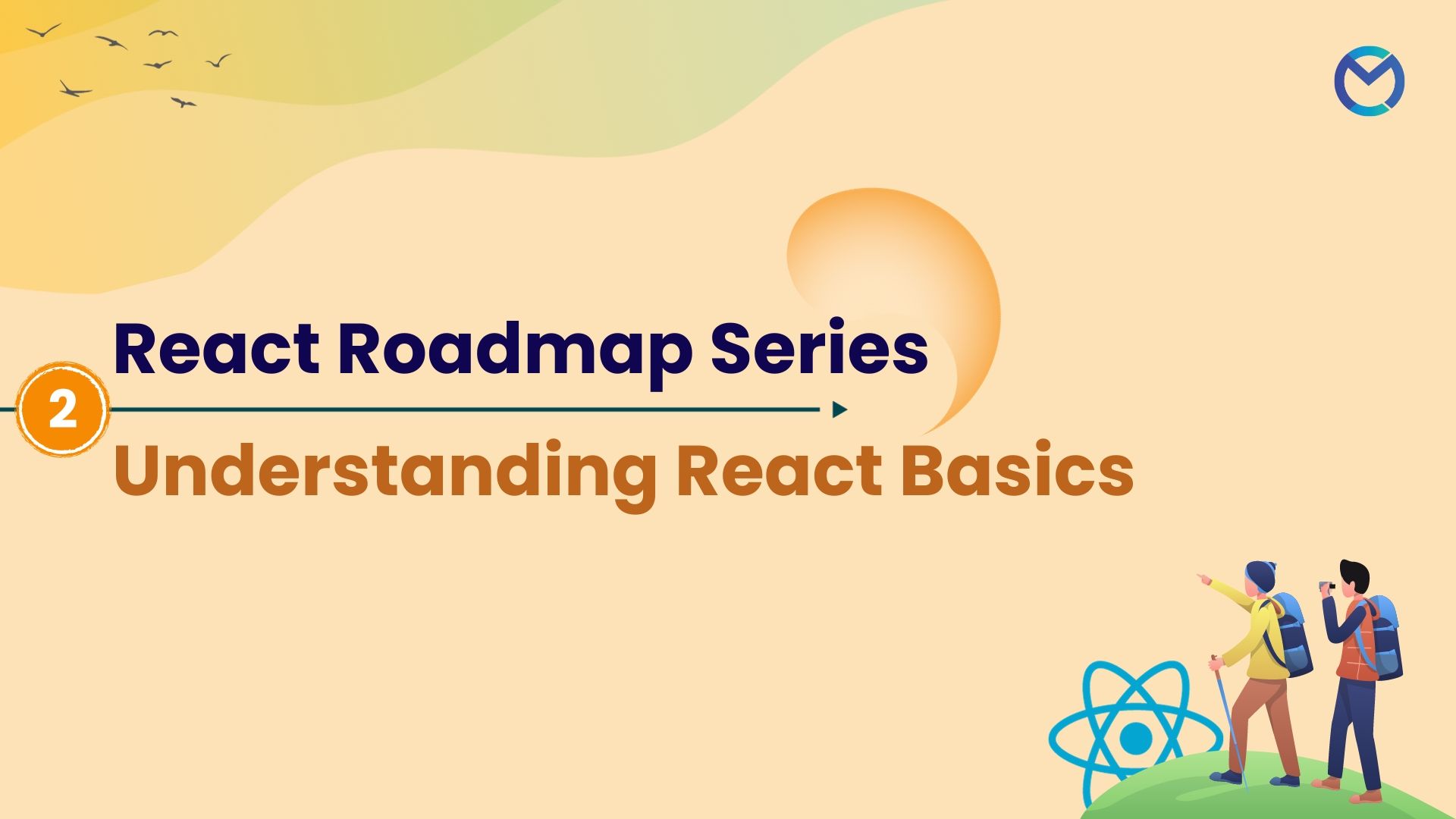
Welcome to the captivating world of React! If you're taking your first steps into web development, you've probably heard of React, a JavaScript library that's become the go-to choice for building modern and dynamic user interfaces. In this beginner-friendly blog, we'll unravel the fundamentals of React, focusing on key concepts that form the backbone of this powerful library.
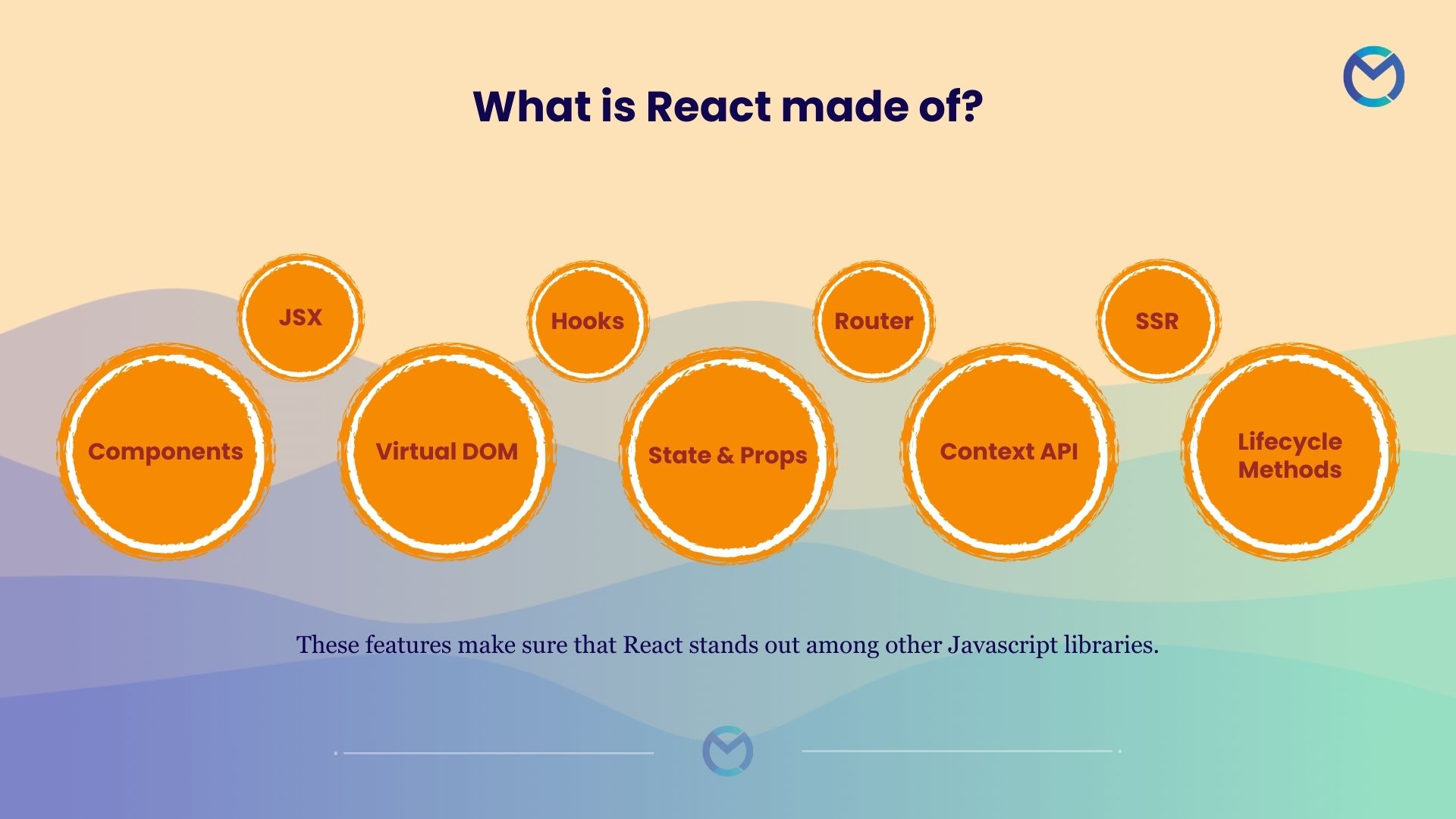
Components
At the heart of React there are components – reusable, self-contained building blocks that encapsulate a piece of the user interface. Components can be as simple as a button or as complex as an entire page. Understanding how to create and use components is fundamental to mastering React.
Types of Components
Class Components
Functional Components
As of React 16.8 and later, functional components with Hooks are the recommended choice for most scenarios. They offer a more concise and modern approach to building components. Class components, while still valid, are considered legacy in comparison. So, let's not dive deep into it. The basic syntax of a functional component looks as given below.
function FunctionalComponentName() {
// ...
}
Virtual DOM
React's use of a Virtual DOM ensures efficient updates to the actual DOM. It minimizes the number of manipulations needed, improving performance by selectively updating only the changed parts.
JSX (JavaScript XML)
JSX is a syntax extension for JavaScript that looks similar to XML or HTML. It allows developers to write UI elements in a syntax that closely resembles HTML within their JavaScript code. JSX is later converted to JavaScript.
import React from 'react';
export default function Portfolio() {
const showAlert = () => {
alert('Button Clicked!');
};
return (
<div>
<h1>React Alert Example</h1>
<button onClick={showAlert}>Click Me</button>
</div>
);
};
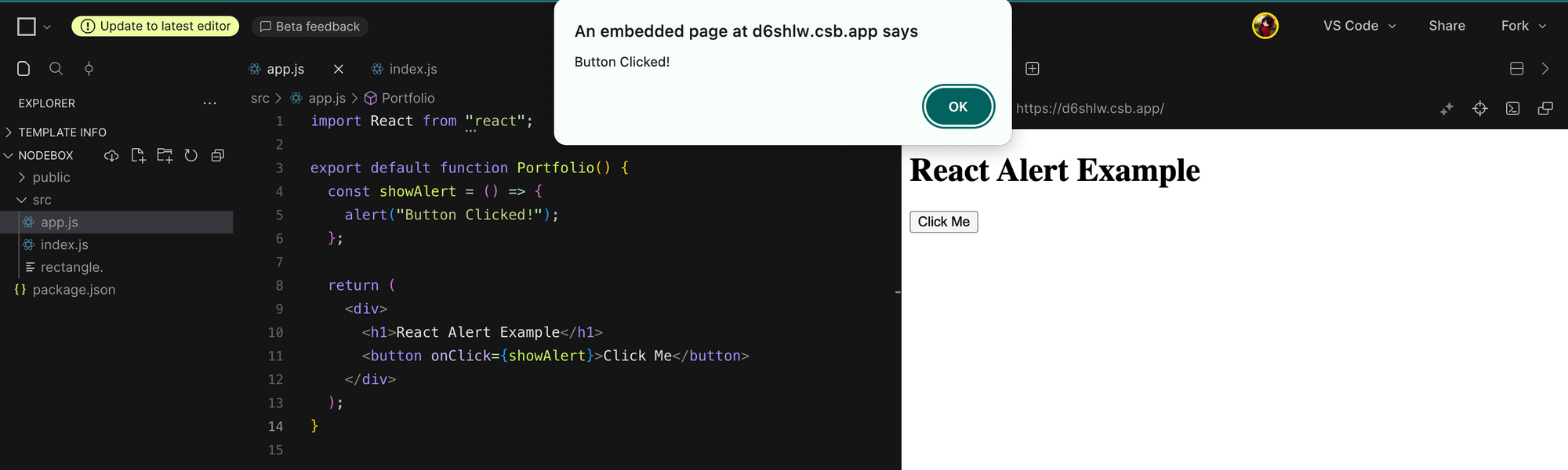
Hooks
Hooks allow functional components to use state and lifecycle features previously available only in class components. Hooks simplify state management and enable the use of side effects within functional components.
To learn more about React Hooks, refer to my blog specially dedicated to it https://blogs.mulecraft.in/the-power-of-react-hooks/
State & Props
In React, state and props are fundamental concepts that enable components to manage and share data.
State is a JavaScript object that represents the current condition or data of a component. It is mutable and can be updated using the setState method.
import React, { useState } from "react";
export default function Counter() {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Add Count</button>
</div>
);}
In this code, we have invoked the increment function during button click, which calls the setCount() function. As we have set the initial state of count as 0 using useState(0) declaration, every button click will add 1 to the existing count value.
Here, useState is a Hook.
Props (short for properties) are a mechanism for passing data from a parent component to a child component. They are immutable and cannot be modified within the child component.
import React, { useState } from "react";
export default function PrintUser({ username }) {
return (
<div>
<p>Current User: {username}</p>
</div>
);}
Here, username is the props and its value can be passed as shown below.
<PrintUser username={"Shan"} />
The component that passes value to the PrintUser child component is the parent.
These concepts are foundational to React's component-based architecture and play a crucial role in building dynamic and reusable UIs.
Router
React Router is a library for handling navigation and routing in React applications. It allows you to build single-page applications with multiple views, manage browser history and navigate between different components without triggering a full page reload.
//Installation Command
npm install react-router-dom
# or
yarn add react-router-dom
import React from "react";
import { BrowserRouter as Router, Route, Routes } from "react-router-dom";
import HomePage from "./pages/HomePage";
export default function App() {
return (
<Router>
<Routes>
<Route path={"/home"} element={<HomePage />} />
</Routes>
</Router>
);}
Here, for the path /home, the HomePage Component is rendered.
Context API
React Context API is a mechanism for sharing state between components without having to pass props through every level of the component tree. It's particularly useful when dealing with global state or when multiple components need access to the same data.
We will discuss about it in detail in the upcoming blogs.
SSR
Server-Side Rendering (SSR) in React is a technique where the initial rendering of a React application occurs on the server instead of the client. This allows the server to generate the HTML content and send it to the client, improving the initial load performance and search engine optimization.
Benefits of SSR
- Improved Initial Load Performance
SSR reduces the time it takes for a user to see the initial content because the server sends pre-rendered HTML. - SEO (Search Engine Optimization
Search engines can easily crawl and index content since it's present in the initial HTML.
Considerations
SSR can add complexity to your application, especially when dealing with client-side state management and asynchronous operations.
For SSR in React, you need a server environment that supports running JavaScript on the server, such as Node.js.
After the initial load, React takes over on the client side, and the client rehydrates the application to make it fully interactive.
Lifecycle Methods
React components have lifecycle methods that provide hooks for executing code at different points in the component's life, such as when it is first mounted or when it is about to be unmounted. Examples include componentDidMount, componentDidUpdate and componentWillUnmount.
As we are going to work with functional components, we are not going to dive deep into this.
To be continued
As you embark on your journey to master React, remember that continual practice, exploration of advanced concepts and staying updated with the vibrant React community will further enhance your skills.
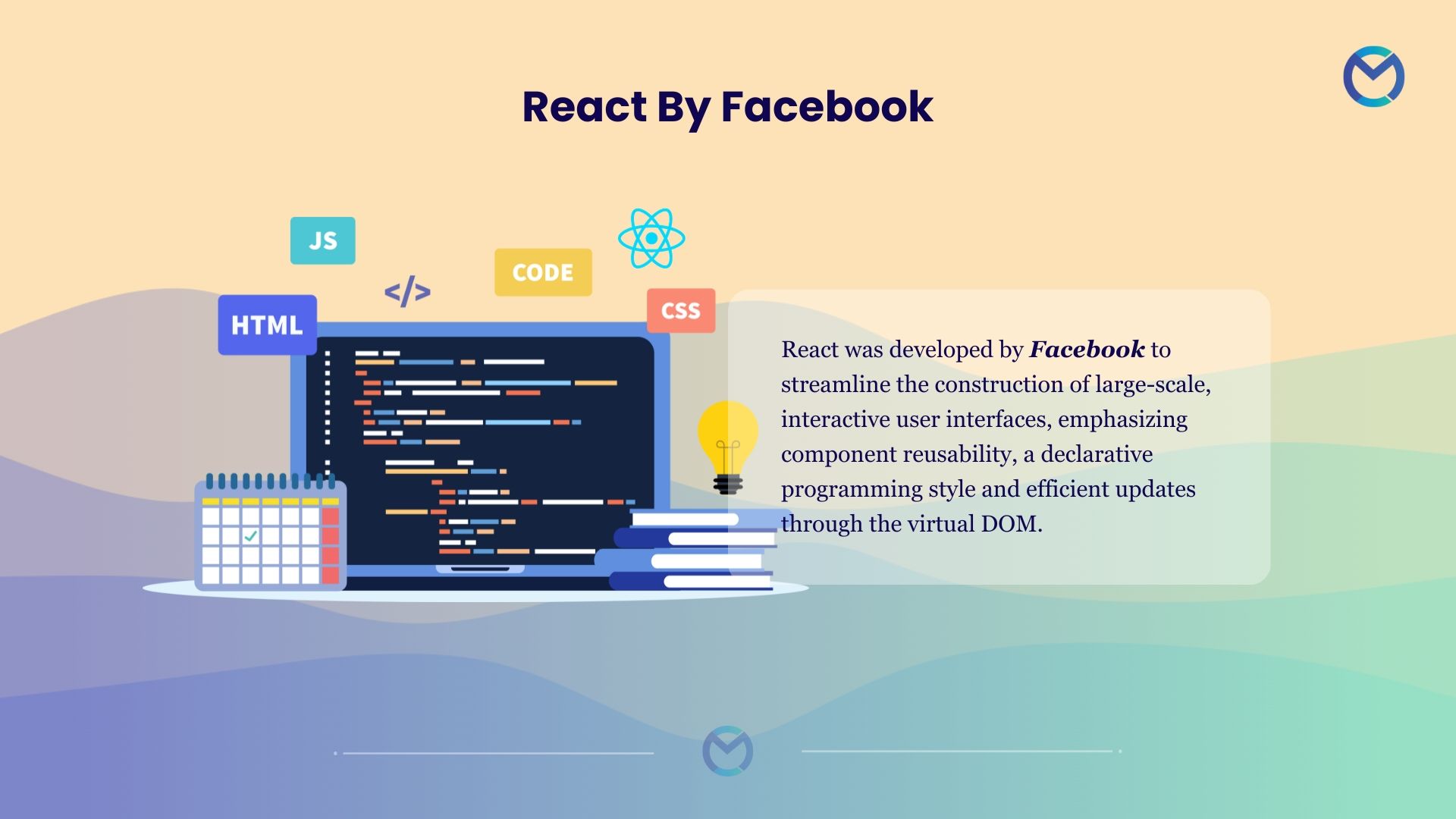
React's flexibility and scalability make it a versatile library, suitable for both small projects and large-scale applications. Whether you're a beginner taking your first steps or an experienced developer expanding your toolkit, mastering React basics is an invaluable investment in your web development skills.
Let us meet at our next stop where we are going to build our first React app and explore more about React Router. Stay connected!